The Art of Debugging: Mastering the Bug Hunt, One Error at a Time
This article talks about the art of debugging: strategies, techniques, and those precious nuggets of wisdom from industry veterans, all served with a side of humor.
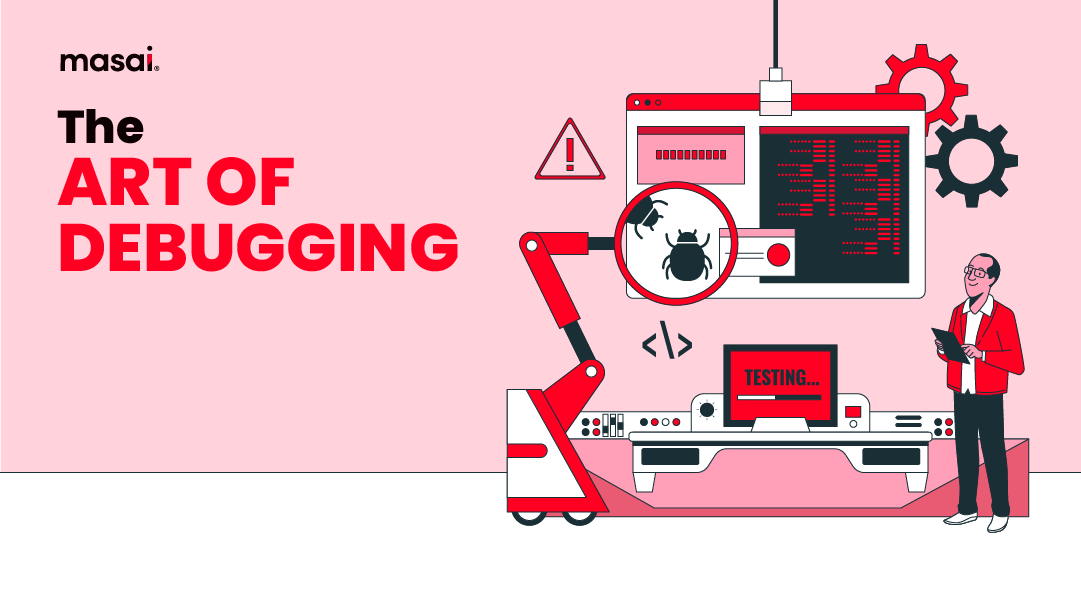
Let’s explore the mastery that is debugging. Get the inside scoop on industry experts' strategies and techniques and some of their precious nuggets of wisdom.
Greetings, code whisperers! Or should I say, code "debuggers"? Before you sigh and click away, let me assure you, this article is not about introducing you to another tedious debugging tool that might make your eyes glaze over. Nope, we're here to talk about the art of debugging: strategies, techniques, and those precious nuggets of wisdom from industry veterans, all served with a side of humor.
The Joy of Error Messages (Yes, You Read That Right!)
Ah, the dreaded red text. The language might change from Python to JavaScript, from C++ to Ruby, but the disappointment is universal.
But hey, look at the bright side! Error messages are the breadcrumbs left by your code, helping you trace your way back to the pesky bug. Celebrate those breadcrumbs because, without them, you'd be in a maze with no way out.
Remember, the first rule of Debugging Club (Yes, it's a thing now) is to read the error message. Sounds obvious? Well, you'd be surprised at how many developers panic at the sight of an error and start a frantic search for a solution without understanding what the problem is.
What is debugging?
Bugs in software development can disrupt the intended behavior, resulting in glitches, errors, or even system failures. To ensure a seamless software experience and swift issue resolution, we offer comprehensive support packages.
To address these challenges, we employ the process of 'debugging,' which involves a meticulous examination of the code and its execution to identify and resolve issues. Debugging empowers developers to gain a deep understanding of program behavior at various stages, allowing them to uncover and rectify flaws.
Consider a complex machine filled with gears, levers, and switches designed to make your favorite ice cream automatically. However, glitches can lead to strange noises, incorrect flavors, or even breakdowns. Debugging, much like detective work, entails dissecting the machine, observing the interactions of its components, and identifying the root problem. By repairing or replacing faulty parts, the machine – or software – can operate smoothly once more.
Similarly, computer programs resemble intricate machines with code, functions, and variables. Unexpected behavior or incorrect results can occur. Debugging involves analyzing these components, correcting problematic code, and ensuring that everything functions as intended.
The Art of Asking "What if...?"
A successful debugging session often starts with a healthy dose of curiosity. Don't just stare at the error message or the faulty output. Instead, start asking "What if...?" questions. "What if this variable has the wrong value?" "What if this function isn't returning what I expect?" "What if this condition never becomes true?" You'd be surprised how many bugs you can squash just by being a little more inquisitive.
Talk to the Duck, No Really
The next time you're stuck, try explaining your code to a rubber duck. This is not a joke, it's an actual technique known as 'Rubber Duck Debugging'. The idea is that by trying to explain your code to an inanimate object (like a rubber duck), you can often spot the error or come up with the solution. It's a bit like having an audience helps you perform better.
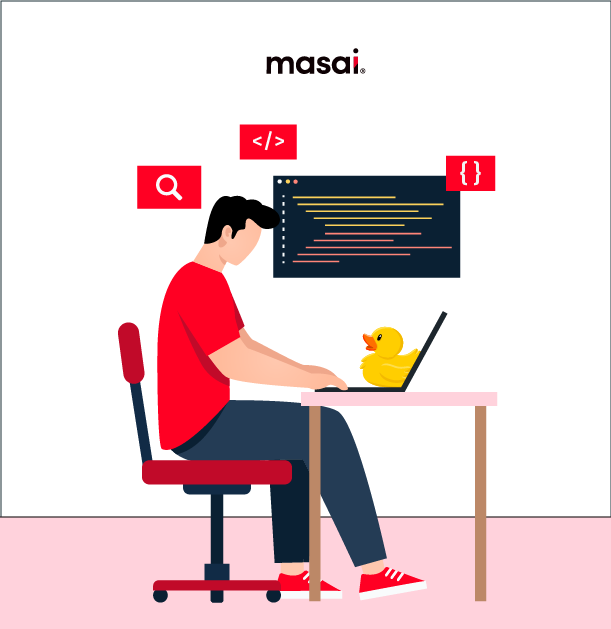
The duck is a patient listener, and no, it won't judge you if your code looks like spaghetti.
Channel Your Inner Sherlock
"Debugging is like being the detective in a crime movie where you are also the murderer." - Filipe Fortes
— Programming Wisdom (@CodeWisdom) August 16, 2017
This gem of a quote from Filipe Fortes, a renowned software engineer, sums up the essence of debugging. Like a seasoned detective, you need to trace the steps of the perpetrator (which is you, remember?), observe patterns, and finally nab the culprit.
The 'divide and conquer' strategy can be your trusty magnifying glass. Break down your code into smaller parts, run them separately, and see where things go south. This way, you can isolate the problem area and focus your efforts where they're needed most.
Making a Bug Appear
Sometimes, bugs are like elusive magicians, appearing and disappearing at their own whims. These intermittent bugs can be a nightmare to debug. The strategy here is to create a reliable way to reproduce the bug.
This might involve setting up specific data, triggering certain actions in a particular order, or even simulating conditions like a slow network or low memory. Once you can make the bug appear consistently, you're halfway to squashing it!
Binary Search Debugging
Remember the 'divide and conquer' strategy we talked about earlier? Well, binary search debugging is an extension of that. If you're dealing with a big chunk of code or a long list of changes, start in the middle. If the problem exists in the middle, the bug is in the first half. If not, it's in the second half. Keep dividing the code or the changes in half until you find the culprit. It's like playing a game of 'hot and cold', but with your code. (More on binary search debugging here.)
Debugging by Sleep
This one might sound a bit odd, but trust me, it works. It's not uncommon to be stuck on a bug for hours, feeling like you're going in circles. When that happens, take a break. Better yet, sleep on it. This isn't just about taking a rest. It's about letting your subconscious mind take a crack at the problem. You'd be amazed at how often you'll wake up with a fresh perspective or even the solution!
Remember, debugging isn't just a science, it's an art. It's not always about finding the quickest solution, but about understanding the problem, exploring different approaches, and learning something new in the process. So, the next time you're faced with a tricky bug, don't despair. Embrace the challenge, enjoy the journey, and celebrate each victory, no matter how small. Happy debugging!
An Ounce of Prevention…
According to Linus Torvalds, the mastermind behind Linux,
"Good programmers know what to write. Great ones know what to rewrite (and reuse)."
This suggests that writing clean, maintainable code can prevent bugs from popping up in the first place. So, invest time in understanding best coding practices. Write meaningful comments, keep your code DRY (Don't Repeat Yourself), and use version control. These practices will make your life a lot easier when you inevitably have to debug your code.
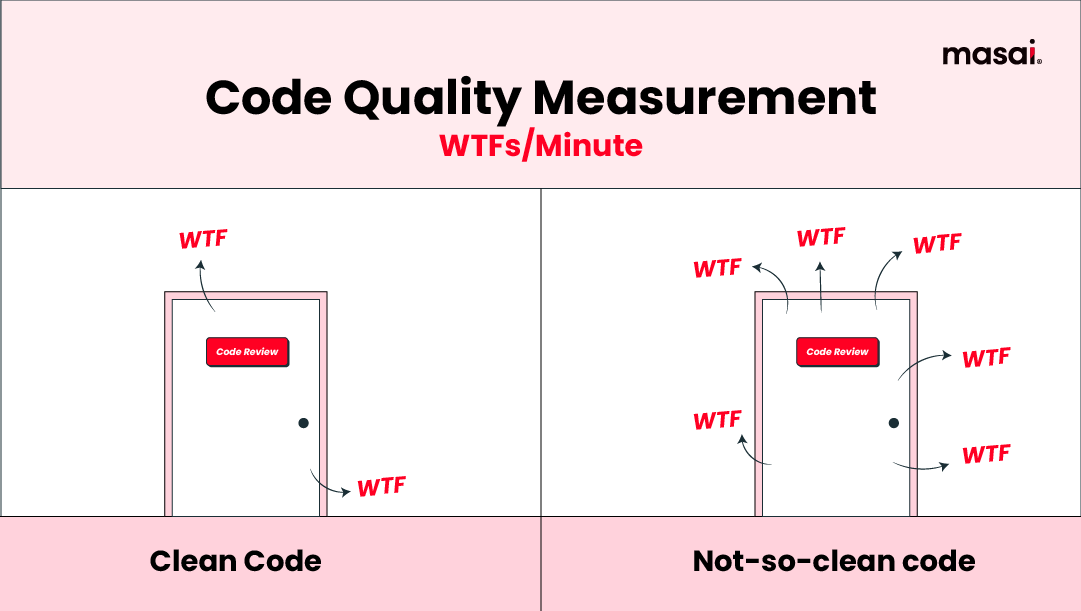
Use Your Tools Wisely
While the art of debugging relies heavily on logic, intuition, and a keen eye, having the right tools in your arsenal can make a world of difference. Be it the humble print() statement, or more sophisticated tools like debuggers in your IDE, use them to your advantage. The key is not to become reliant on a single tool but to have a diverse range of weapons at your disposal.
Some modern debuggers even allow you to move back and forth in your code execution. Tools like the Replay Debugger for Visual Studio Code or rr for C++ let you record your program execution and replay it as many times as you want. This is extremely useful for understanding complex bugs that involve many steps or happen over a long period.
Debugging Is Not a Solo Sport
While it may be tempting to see debugging as a battle of wits between you and your code, don't forget the value of a fresh pair of eyes. There's a reason why code reviews and pair programming are popular in the industry.
They allow you to get a different perspective on your code, catch bugs you might have overlooked, and learn from others' strategies. Remember, programming is as much a social activity as it is a technical one.
Breakpoints
Breakpoints, in the context of software development, serve as markers or designated points inserted by developers within a program's source code. These markers serve the purpose of momentarily suspending the program's execution when reached, thereby affording developers the opportunity to inspect various aspects such as the program's state and variables. The utility of breakpoints lies in their role as a fundamental tool for the debugging and troubleshooting of software applications.
To draw an analogy, one can liken breakpoints to predetermined inspection stations along a factory's production line. Just as these inspection points temporarily halt production when a potential issue arises, breakpoints effectively pause program execution when an anomaly is detected, enabling developers to closely scrutinize the program and pinpoint the underlying problem.
Divide and Conquer
The "Divide and Conquer" debugging strategy is a valuable approach, particularly when dealing with extensive or intricate codebases. It involves breaking down the problem into smaller, more manageable components to facilitate the identification and resolution of issues. Here's an expanded explanation of this strategy:
When confronted with a large or intricate codebase, the "Divide and Conquer" strategy becomes an indispensable tool for developers. Instead of attempting to tackle the entire codebase as a monolithic entity, this approach advocates for breaking down the problem into smaller, more digestible parts.
One effective method is to selectively comment out sections of the code that you suspect might be related to the issue at hand. By temporarily deactivating specific code segments, you can isolate the problem to determine whether it resides within that particular portion or if it is indicative of a broader issue.
Additionally, creating minimal test cases can further assist in isolating the problem. These simplified scenarios involve extracting only the essential elements of the code necessary to reproduce the issue. By doing so, you can focus your debugging efforts on a highly controlled environment, making it easier to identify the root cause of the problem.
Wrapping Up
In the words of the legendary software engineer, Edsger W. Dijkstra,
"If debugging is the process of removing software bugs, then programming must be the process of putting them in."
Let's face it, bugs are an inherent part of coding. They're not a sign of incompetence but an opportunity for learning and growth.
So next time you encounter a bug, instead of groaning in frustration, take a deep breath, put on your detective hat, and start unraveling the mystery. Who knows, you might even start enjoying the thrill of the chase!
In the end, debugging is not just about squashing bugs; it's about becoming a better coder. It's about understanding your code profoundly, refining your thought process, and honing your problem-solving skills.
So, keep coding, keep debugging, and remember to have fun along the way. Because as we all know, a day without laughter (or a bug) is a day wasted. Happy debugging, folks!
More resources:
Unleashing the Future: How AI is Transforming Software Development
How does Recursion work? Recursion vs Iteration
8 Transforming Productivity Tips for Developers