Applications of Array Explained
Even if you look around, arrays are one of the most common structures you’ll get. From assembly lines to the contact list on your phone, from egg cartons to online ticket booking portals, the list is endless.
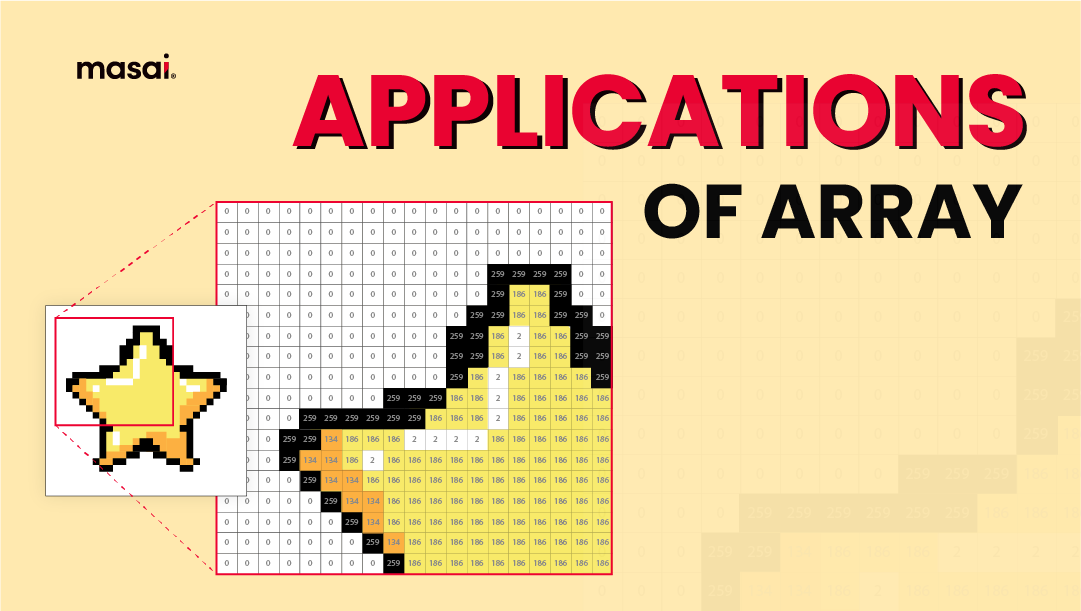
Even if you look around, arrays are one of the most common structures you’ll get. From assembly lines to the contact list on your phone, from egg cartons to online ticket booking portals, the applications of arrays are endless.
If you’re a programming student, array data structure is one of the first concepts you’ll get to learn in data structures & algorithms. It’s that prevalent and that important.
To start with the technical definition:
“Arrays are a type of data structure in which elements of the same data type are stored in contiguous memory locations.”
As we know, data structures are nothing but different means to store data in a structured manner. An array is one of those means.
But it’s likely that you don’t understand the definition of the array if you’re a beginner. So, we’ll take a few examples here-
You can think of an array as a row of boxes, each containing an item, and all the items are of the same type. If the first box contains an egg, all the other boxes must contain eggs, too for it to be an array. ‘Same data type’ remember?
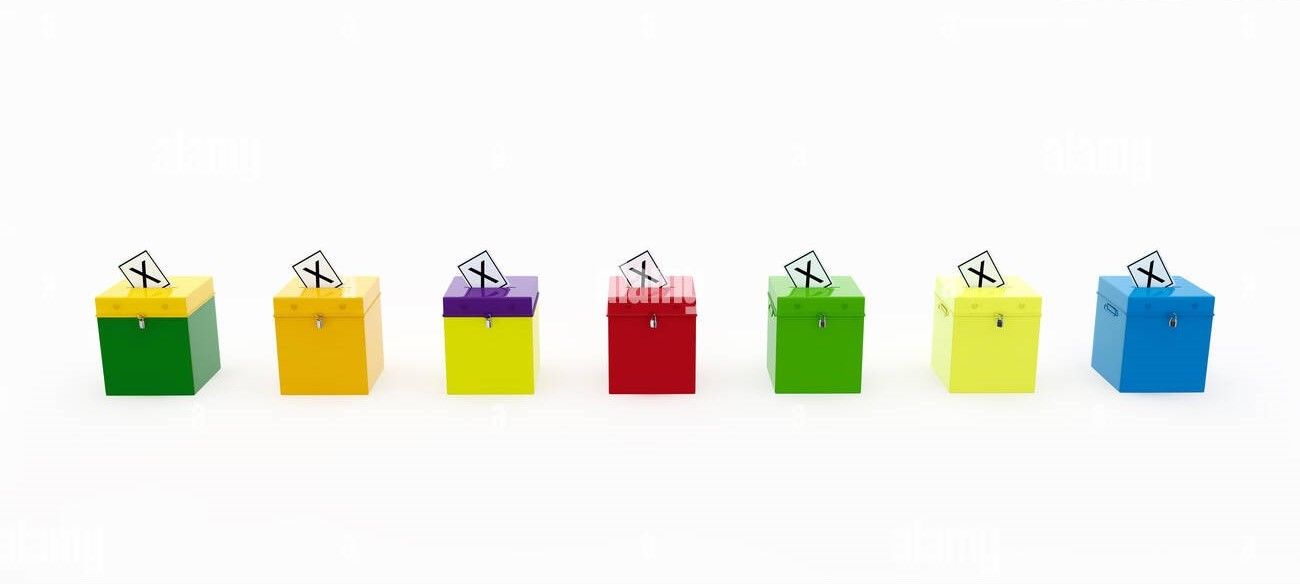
Now, we assign each egg a positive numerical value known as an index, which correlates to its position. This would be an example of a one-dimensional array.
The difference is that the eggs will be replaced with elements such as integers and strings.
It should also be noted that array indices normally begin with a value of 0. (starting from 0 instead of 1 is a repeating theme in programming).
As we have discussed in our previous article[1], arrays can be both one-dimensional and multi-dimensional. A multi-dimensional array (2D or 3D array) can be simply considered an array of arrays. A 2D array can be picturized as a table made of rows and columns like a spreadsheet or a chessboard. And a 3D array is simply a collection of 2D arrays.
Basic Terms of An Array Data Structure
- In arrays, an element refers to a particular item that is stored.
- Each element carries an index - a location with respect to a base value.
- The base value is the memory location of the first element of the array.
- We simply add offsets to this value which makes it easier for us to use the reference and identify items.
- Array length – the number of elements an array can store is defined as the length of the array. It is the total space allocated in memory while declaring an array.
Here are a few important properties of an array:
- Each entry in an array has the same data type and size, which is 4 bytes.
- The array's elements are stored in contiguous memory regions, with the first element placed in the smallest memory address.
- Elements of the array can be accessed at random because we can define the address of each element using the specified base address and data element size.
- Arrays make good use of computer addressing logic because the memory in most modern computers and external storage devices is a one-dimensional array of words.
Before jumping onto the applications of arrays, let’s understand why we use arrays. What benefits does it bring to the table? (except being a table itself)
Why do we need arrays?
- It is simpler to sort and search for a value in an array
- Arrays are ideal for processing many values with ease
- Arrays are useful for storing a variety of values in a single variable. Most applications of of arrays in computer programming necessitate keeping a significant amount of data of a similar type. To hold this much data, we must define a large number of variables. While writing the programs, it would be quite tough to remember the names of all the variables. Instead of naming each variable a different name, it’s simpler to build an array and store all of the elements within it.
Applications of Arrays
We’ve already discussed everything about arrays in our previous article. Here we’ll try to understand the applications of arrays in the programming world using proper examples and images. Let's get started:
1. Implementation of Stacks and Queues
Arrays can be used to implement stack[2] and queue[3] data structures. Both stacks and queues are linear data structures having a wide range of applications. Unlike linked lists[4], arrays are easier to implement the stacks and queues.
Let’s see how different operations can be implemented on a stack using an array-
Adding an element onto the stack
The push operation (the process of adding an element on the top of a stack) consists of two phases:
- We increment the top variable so it can now refer to the next memory location
- Then we add a new element at the position of the incremented top.
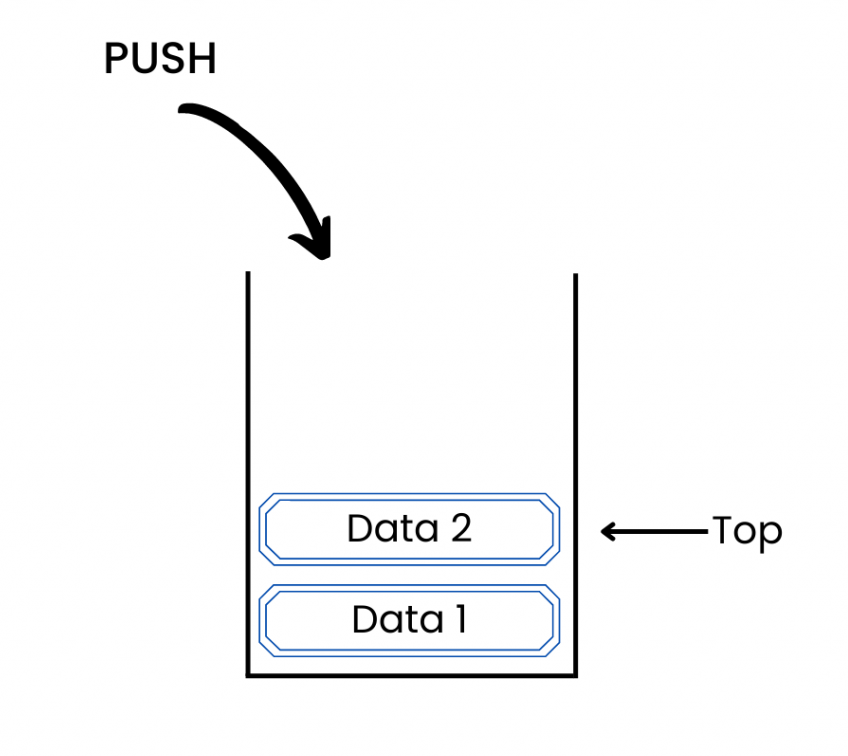
When we try to put an element into a totally loaded stack, the stack overflows; thus, our main function must always be to avoid stack overflow.
Here’s the algorithm for the Push operation in C language-
void push (int val,int n) //n is size of the stack
{
if (top == n )
printf("\n Overflow");
else
{
top = top +1;
stack[top] = val;
}
}
Deleting an element from the stack
The process of removing an element from the stack is called the Pop operation. While implementing the Pop operation using arrays, the data element is not actually removed from the stack, instead, the ‘top’ variable is decremented to a lower position to refer to the next element below.
Meanwhile, the stack's topmost component is stored in another variable, which is subsequently decremented by one. As a result, the procedure returns the previously saved removed value from another variable.
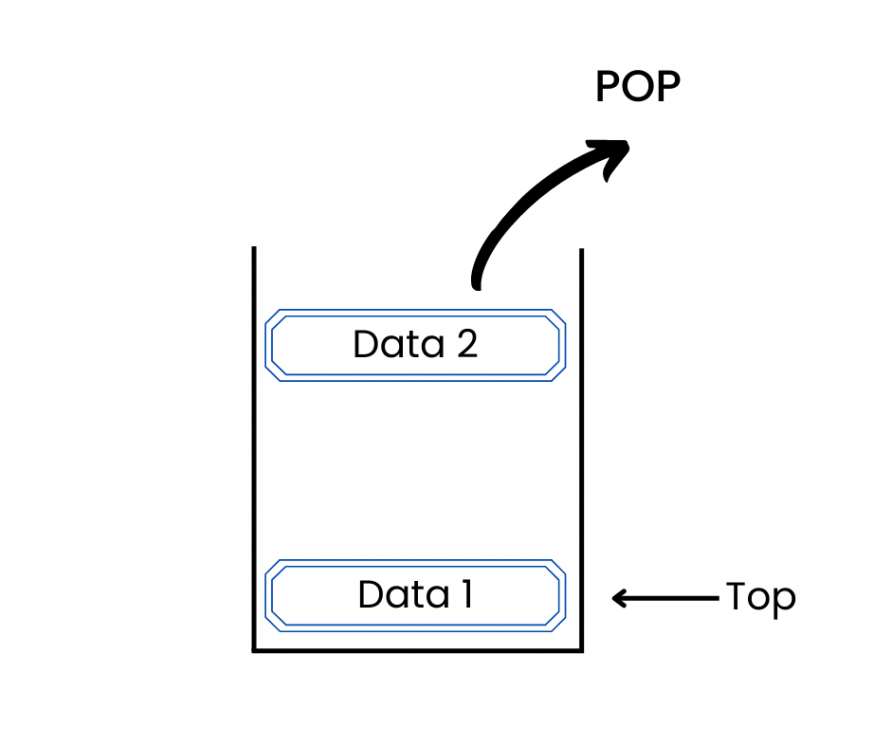
When we try to delete an element from an already empty stack, we encounter the underflow condition.
Here’s the algorithm for the Pop operation in C language:
int pop ()
{
if(top == -1)
{
printf("Underflow");
return 0;
}
else
{
return stack[top - - ];
}
}
Similarly, arrays can be used to form a queue and implement different operations on it. (Here's the array implementation of a queue in detail.[5])
2. Implementation of other data structures
Arrays are also used to implement other data structures such as lists, heaps, hash tables, strings, and VLists. Implementations of data structures using arrays are comparatively simple and space-efficient, require lesser space overhead but may have poor space complexity, particularly when modified/updated, compared to tree-based data structures[6].
3. CPU Scheduling
For those who don’t know, CPU scheduling is the process by which the CPU decides the order and manner in which multiple tasks would be executed when it’s asked to perform multiple tasks. Arrays can be a handy data structure to contain the list of processes that need to be scheduled for CPUs that we need to keep track of.
- In Linux CPU scheduling, a runnable job is considered executable if it has not spent all of the time available in its allotted time quantum. These jobs are placed in an active array, which is indexed by priority.
- When a job’s time slice expires, it is moved to an expired array. As part of the transfer, the priority of the tasks may be re-assigned.
- The two arrays are exchanged when the active array gets empty.
- Runqueue structures are used to store these arrays. Each processor on a multiprocessor computer has its own scheduler and runqueue.
4. Processing an Image
Do you know how computers process an image? Well, definitely not like we do.
Let’s take this image as an example.
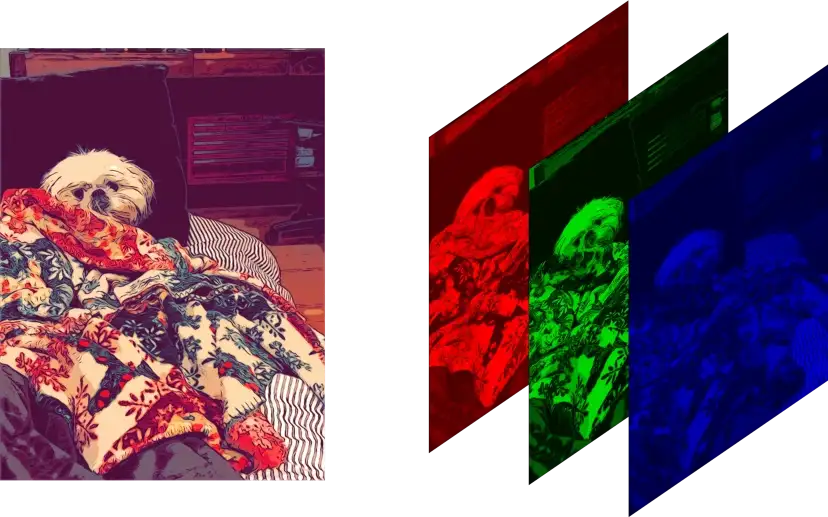
On the left is the original image. When a computer scans or retrieves this image, it will be broken down into three different channels- red, green, and blue as shown on the right. These are the three colours that compose white light.
Now as we all know, computers only understand data in the form of numbers. These channels are then converted into a three-dimensional array as shown in the image below.
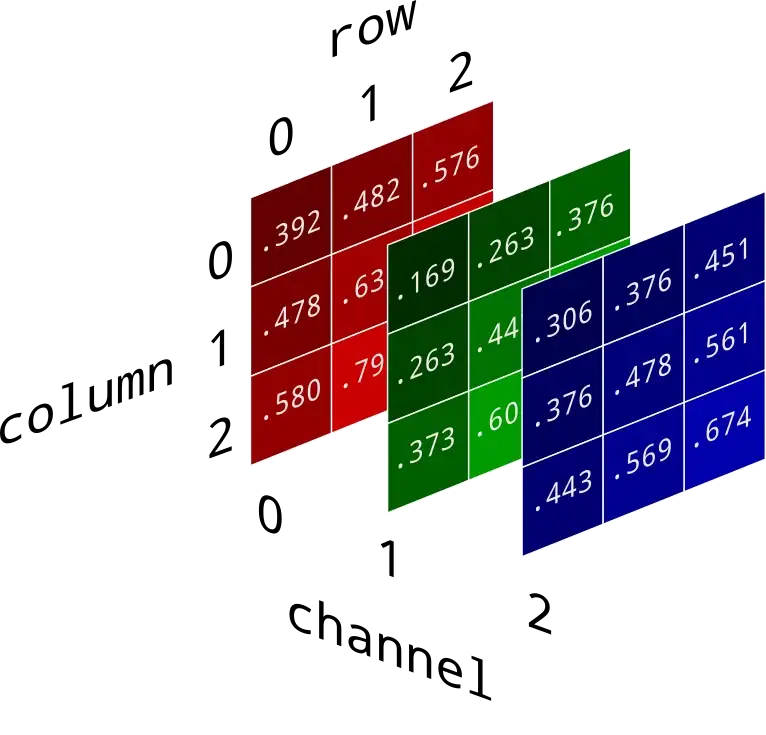
Each array cell corresponds to a pixel in the image. Meaning that the dimensions of the converted array will always be the same as the image’s resolution. Thus, a colour image with a resolution (800*600) will be divided into three 2D arrays containing the same number of cells. The values contained in each array cell represent the channel intensity for the corresponding pixel.
And this is how arrays are used for image processing.
5. Implementation of complete binary trees
In complete binary trees, every level must be filled, and all the leaf elements lean towards the left. While implementing the tree, an array can efficiently store the tree's data values by storing each data value in the array position that corresponds to that node's position inside the tree.
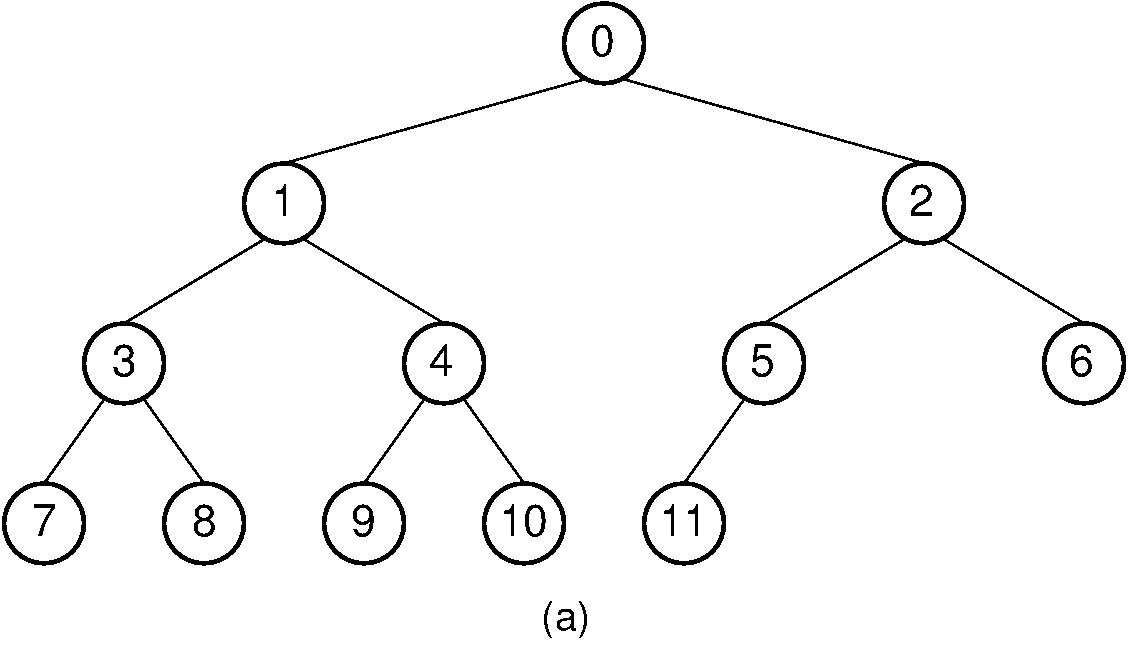
The below table shows the array indices for each node's children, parent, and siblings.

When you look at the table, you should see a pattern in the placements of a node's relatives within the array. We can derive simple formulas to locate each relative of a node X from X’s index.
No pointers are needed to reach a node's left or right child. This indicates that if the array is chosen to be of size n for a tree with n nodes, there is no overhead to the array implementation.
And thus, arrays can be a better approach to implementing tree data structure, when compared to pointers.
Here are a few other important applications of arrays:
- Arrays can also be used to implement lookup. The lookup table is an array in and of itself that stores pre-calculated values that save the calculation time required to compute the given data.
- Since an array can maintain multiple variables under a single name, it can be used to store large amounts of data. It avoids the confusion of using multiple variables.
- The applications of arrays can also be seen in Matrix operations[7]. Many databases (small and large) are made up of one-dimensional and multi-dimensional arrays.
- Matrix operations[7] can be implemented using arrays. Many databases (small and large) are made up of one-dimensional and multi-dimensional arrays.
- We can use arrays to determine the partial or complete flow in the code. It can be termed as an alternative to multiple IF statements. They are used in conjunction with an interpreter and are referred to as control tables in this context. The array values regulate the flow of control in this interpreter.
- Speech processing is a field where the applications of arrays are important since every speech signal is an array. Microphone arrays[8] can be used to enable distant-talking engagement in Automatic Speech Recognition (ASR) systems. Their beam-forming abilities are used to improve the spoken message while reducing the unwanted impact of environmental noise and reverberation.
- Cryptography is another great example when it comes to applications of arrays. Arrays are widely used in data transformations for encryption and decryption.
- We can also see the applications of arrays in machine learning and data science. Arrays store feature vectors and data sets and play a significant role in training models and making predictions.
Wrapping Up
Arrays, being the most primitive data structure, obviously have numerous applications. Even if you look around, arrays are one of the most common structures you’ll get. From assembly lines to contact lists on your phone, from egg cartons to online ticket booking portals, the list is endless.
Hope you’ve understood the applications and gotten new perspectives on arrays after reading this article, whether you’re a beginner or a professional.
We at Masai have always kept our primary focus on training students in DSA[9] in our part-time and full-time courses in full-stack web development. Students get to solve daily DSA problems and interview questions throughout the 35-week course.
If you want to become a full-stack developer and work in top companies, take the first step and check out these courses.
Cheers!
Resources:
- Array Data Structure- Explained with Examples
- Stack Data Structure- Explained with Examples
- Queue Data Structure- Types, Applications, JavaScript Implementation
- Linked List in Data Structures - Explained with Examples
- Array Implementation of Queue
- Tree Data Structure- Types, Operations, Applications
- Matrix Operations using arrays
- Microphone array speech processing
- Data structures & algorithms- Explained with Examples
FAQs
What are some real-time applications of SAS temporary arrays?
Real-time SAS temporary arrays find use in streaming data analysis, financial modeling, sensor data processing, and real-time decision-making, enabling efficient data manipulation and analysis on the fly.
What is an application of array in data structures?
Arrays are widely used in data structures for tasks like storing and accessing elements in a contiguous memory block, supporting efficient indexing, and facilitating iterative operations in algorithms.
What are the real world applications of data structures like arrays, structures, linked lists, tress, graphs, heaps, sorting?
Arrays are used to store data efficiently, for example, Tabulating data, image processing. Structures are used to organize complex data and database records. Linked lists help in task scheduling and memory management. Trees organize file systems and hierarchical data. Graphs model relationships such as social networks and navigation systems. Heaps optimize priority queues. Sorting enhances data retrieval as in the case of database indexing and search algorithms.