Understanding React Components: Functional vs. Class Components
Dive into the realm of React components. Explore different types, learn creation techniques, and follow best practices for seamless UI development.
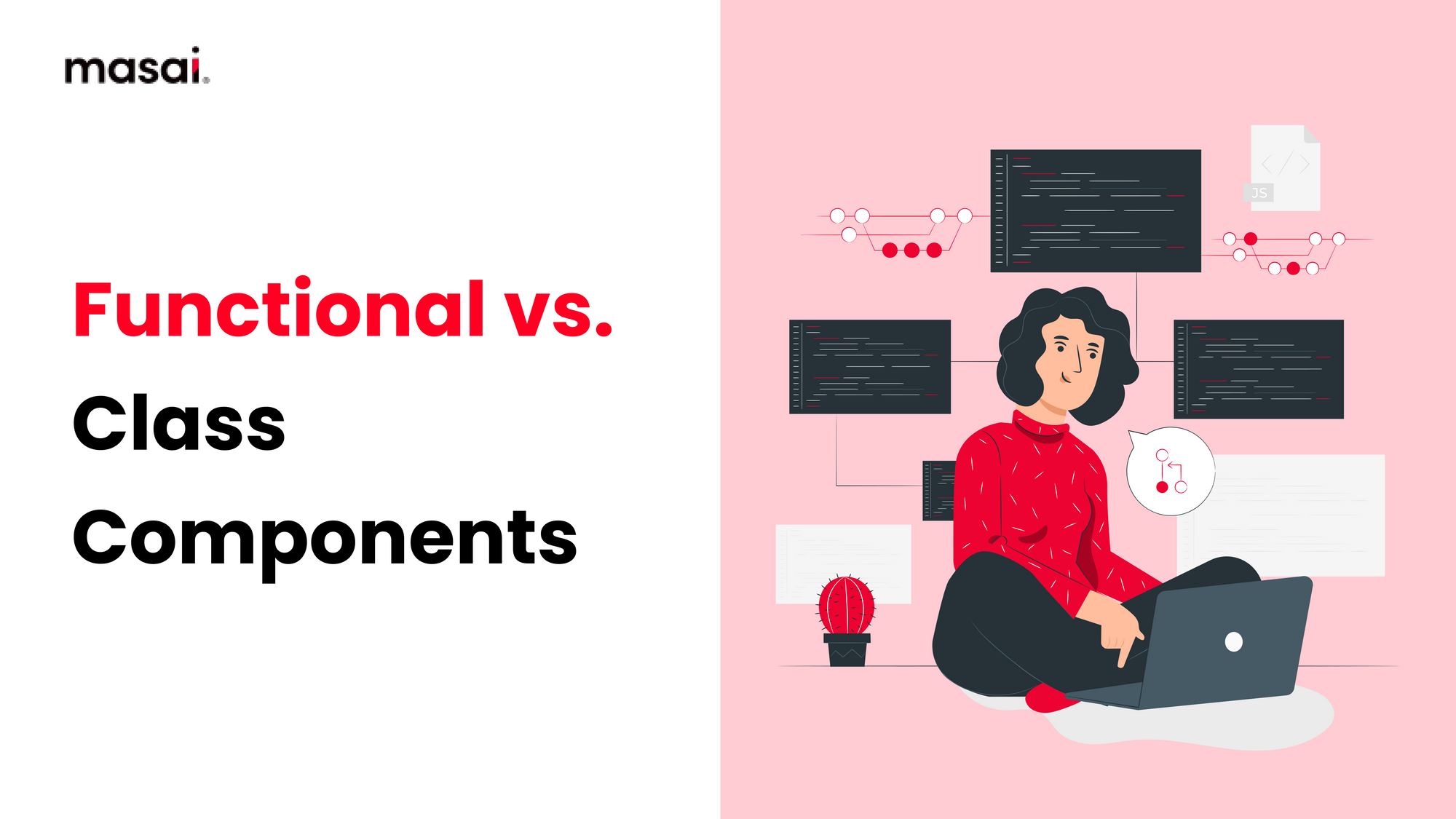
Introduction
React, the JavaScript library for building user interfaces, has revolutionized web application creation. At the heart of React's architecture lie components, the modular building blocks that enable developers to construct complex UIs with ease. Within the realm of components, there are two main contenders: functional components and class components. In this exploration, we'll delve into the nuances of these two types, uncovering their strengths, weaknesses, and best use cases. By understanding the distinctions between functional and class components, you can make informed decisions that enhance your React development process. Let's embark on this journey to uncover the dynamic interplay between these essential components in the React ecosystem.
The Basics of React Components
React is fundamentally about creating user interfaces by composing several components. A component is a unit of functionality or a UI element, and mixing components to build intricate user interfaces is possible. Like building blocks, React components serve a specific function and can be used repeatedly throughout the program.
Functional Components
Functional components are, as their name suggests, primarily JavaScript functions that produce JSX (JavaScript XML) to define the component's user interface. They have grown in popularity as a result of their simplicity and usability. In essence, a functional component is a pure function that receives props as input and outputs a JSX element to render. With the introduction of hooks, functional components can also manage local states and utilize lifecycle functionalities previously exclusive to class components.
One of the primary advantages of functional components is their readability. They feature a concise syntax that makes code more understandable, reducing the cognitive load for developers. Additionally, functional components are easier to test due to their deterministic nature – given the same inputs, they will always produce the same output.
Class Components
Class components were the original way of creating components in React. They are constructed using JavaScript ES6 classes and provide more advanced features than functional components. Class components are known for using lifecycle methods, allowing React developers to manage component behavior at different stages, such as mounting, updating, and unmounting.
However, class components have a steeper learning curve and can be more verbose than their functional counterparts. The lifecycle methods can also make the component logic harder to follow, especially as the component grows in complexity. Despite these challenges, class components have been a staple in React development and can still be found in many existing codebases.
Comparing Functional and Class Components
As you embark on your React journey, it's crucial to understand the differences between functional and class components, as they can impact your development process and the quality of your code. Let's dive into a comprehensive comparison to help you make informed choices.
Use Cases for Each Component Type
Understanding when to use functional components and when to opt for class components is crucial to making informed architectural decisions in your React projects. Let's explore scenarios where each component type shines.
Functional Components
Functional components are well-suited for projects prioritizing simplicity, readability, and reusability. They are particularly useful for:
- Presentational Components: When dealing with UI elements that primarily render data and don't involve complex logic, functional components offer a concise way to create clean and readable code.
- State Management with Hooks: With the introduction of hooks like useState, functional components can now manage local states without the complexity of class components. This makes them ideal for components with moderate state management requirements.
- Component Testing: Functional components' deterministic nature makes them easier to test, as their behavior is closely tied to their inputs and outputs.
Class Components
While functional components have gained prominence, there are still scenarios where class components hold value:
- Complex Logic and Interactions: When dealing with components that require intricate business logic or complex interactions, class components provide a structured approach through lifecycle methods.
- Legacy Codebases: In projects with existing class components, migrating to functional components might not be practical. Class components allow you to maintain consistency within the codebase.
- Learning and Understanding: For those new to React or transitioning from class-based frameworks, learning and understanding class components can provide valuable insights into the core concepts of React.
Choosing between functional and class components depends on your project's requirements and your team's familiarity with both approaches.
Transitioning from Class to Functional Components
Migrating from class components to functional components is a progressive step in embracing modern React practices. To transition, follow these steps:
- Identify Components: Start by identifying class components that can benefit from the transition.
- Refactor Logic: Rewrite the component's logic using functional components and hooks like useState, useEffect, and others.
- Update Lifecycle Methods: Replace class-based lifecycle methods with corresponding hook equivalents.
- Test Thoroughly: Rigorously test the refactored component to ensure it maintains functionality.
- Iterate and Optimize: Refine your code, focusing on readability and performance.
- Repeat Process: Gradually transition other class components as needed, maintaining a consistent codebase and reaping the benefits of modern React practices.
Conclusion
In the realm of React components, the choice between functional and class components depends on your project's needs and development goals. With their clear syntax and hooks-driven state management, functional components have emerged as the preferred approach for many modern applications. However, class components still hold relevance in scenarios involving complex logic and legacy codebases. By understanding the strengths and weaknesses of each type, you can make informed decisions that enhance code readability, maintainability, and performance. Whether you embrace the elegance of functional components or leverage the familiarity of class components, your React journey is enriched by this nuanced understanding of these essential building blocks.
FAQs
What are React components, and why are they important?
Modular building elements called React components are used to create user interfaces. They enable developers to design sophisticated interfaces by encapsulating particular functions or UI elements.
What distinguishes functional components from class components?
Functional components, valued for their simplicity and clarity, are JavaScript functions that return JSX to create UI. Using ES6 classes, class components can offer more complex features like lifecycle methods. Having an understanding of these differences aids developers in selecting the proper component type.
How do functional and class components handle lifecycle events?
While functional components employ hooks like use effect, class components rely on lifecycle methods. Hooks offer a unified approach to control component behavior, eliminating the need for dispersed techniques.
What are the main benefits of functional elements?
Functional components provide clear syntax, determinism simplifies testing, and hooks simplify state management. They work best for moderate state management and straightforward UI presentation.
In my React project, when should I utilize functional or class components?
Simple, readable, and presentational UI elements are chosen over functional components. Class components are excellent for learning the principles of React as well as for managing complex logic and legacy codebases.