Preparing for Spring Boot Interviews: Common Questions and Tips
Get ready for your Spring Boot interview with our detailed Q&A. Understand key features, microservices, annotations, and more for interview success.
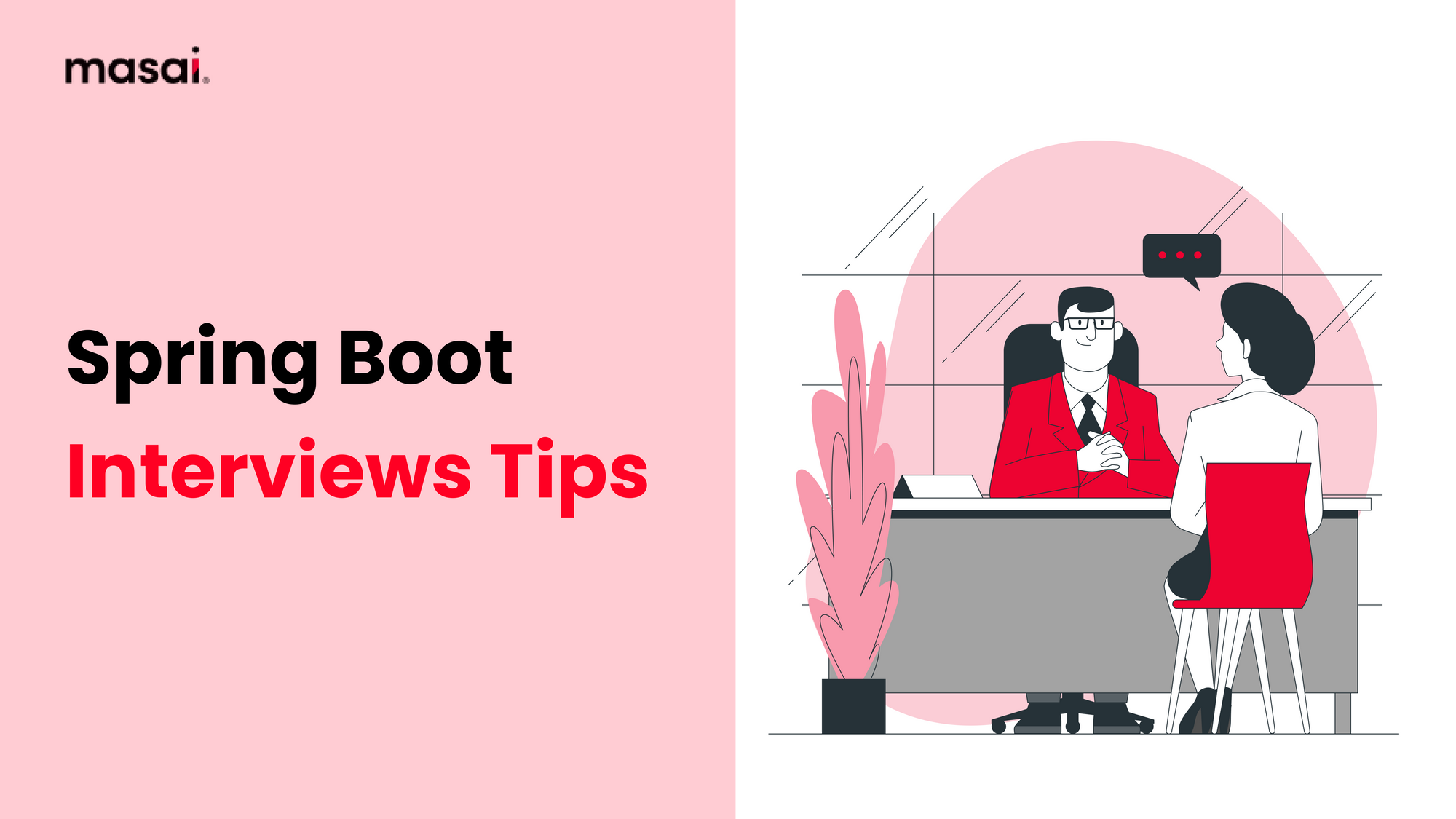
Introduction
In the fast-paced world of software development, staying ahead of the curve is essential. With its ease of use and powerful capabilities, Spring Boot has become a cornerstone of modern application development. As the demand for Spring Boot expertise continues to rise, interviews have become the gateway to exciting career opportunities in this realm. A Spring Boot interview isn't just about demonstrating theoretical knowledge; it's about showcasing practical skills that can make a real impact.
Common Spring Boot Interview Questions
Here are some of the common and frequently asked Spring Boot interview questions with answers for web developers.
1. Question: What is Spring Boot, and why is it popular in the development community?
Spring Boot is an innovative framework built on top of the Spring framework, designed to simplify and accelerate the process of building production-ready applications. It streamlines application setup, configuration, and deployment by providing defaults and conventions. This popularity stems from its ability to reduce development time, eliminate boilerplate code, and promote the use of microservices architecture. Spring Boot's integrated support for technologies like Spring Data, Spring Security, and Spring MVC further enhances its appeal.
2. Question: Explain the concept of Dependency Injection in Spring Boot.
Dependency Injection (DI) is a core principle in Spring Boot that promotes loose coupling between components. In DI, objects are not responsible for creating their dependencies; instead, they receive them from an external source. Spring Boot's Inversion of Control (IoC) container manages the injection of dependencies. This allows for easier testing, maintenance, and scalability of applications. Using annotations like @Autowired, developers can inject required dependencies into their classes.
3. Question: What is Spring MVC, and how does it work in Spring Boot?
Building web apps uses the Model-View-Controller (MVC) design pattern from Spring. Spring MVC facilitates web application development with Spring Boot by offering a clearly defined framework. The Controller controls requests and orchestrates the data flow between the Model and View, whereas the Model represents the data, and the View manages the user experience.
4. Question: How does Spring Boot ensure data persistence?
Spring Boot seamlessly integrates with Java Persistence API (JPA) and Hibernate, enabling efficient data persistence in relational databases. Developers can create, retrieve, update, and delete data from the database by defining entities and their relationships using JPA annotations. Spring Boot's automatic data source configuration and Hibernate's object-relational mapping simplify database operations. With Spring Data JPA repositories, developers can avoid writing boilerplate code for common database operations, making data access more intuitive and efficient.
5. Question: What is Spring Security, and how can it be implemented in Spring Boot applications?
Spring Security is a powerful framework that provides authentication, authorization, and other security features to Spring applications. In Spring Boot, integrating Spring Security is straightforward. Developers can add the spring-boot-starter-security dependency, and Spring Boot takes care of the initial setup. Developers can define who can access various application parts using annotations like @EnableWebSecurity and configuring security rules. This level of security is essential for protecting sensitive data and ensuring a secure user experience.
6. Question: How does Spring Boot handle exception handling?
Spring Boot simplifies exception handling through its built-in error-handling mechanisms. The framework provides a centralized way to handle exceptions globally, ensuring a consistent response format. Developers can create custom exception classes and annotate them with @ResponseStatus to define HTTP status codes. Additionally, by implementing @ControllerAdvice, developers can define global exception handlers to manage different types of exceptions across the application. This approach enhances code readability, maintainability, and user experience by providing meaningful error messages.
7. Question: Explain the concept of AOP (Aspect-Oriented Programming) in Spring Boot.
Aspect-Oriented Programming (AOP) is a programming paradigm that enables modularization of cross-cutting concerns. Spring Boot integrates AOP through AspectJ, allowing developers to separate concerns such as logging, security, and transaction management from the core business logic. Using annotations like @Aspect and @Before, developers can define aspects that intercept method calls and apply additional behavior. This promotes code modularity, reusability, and maintainability while reducing code duplication.
8. Question: How can you secure a REST API in Spring Boot?
Securing a REST API in Spring Boot can be achieved through Spring Security. Using token-based authentication, such as JSON Web Tokens (JWT), developers can ensure that only authorized users can access protected resources. Spring B0oot's integration with Spring Security makes it straightforward to configure security filters, define authentication providers, and control endpoint access. By annotating methods with @PreAuthorize or @RolesAllowed, developers can enforce fine-grained authorization rules, providing a secure API for users.
9. Question: Discuss Spring Boot's support for microservices architecture.
Spring Boot is well-suited for building microservices due to its lightweight nature and modular design. It supports the creation of standalone microservices that can communicate with each other through APIs. Spring Cloud, a set of tools and frameworks, enhances Spring Boot's capabilities for building and deploying microservices.
10. Question: How can you optimize the performance of a Spring Boot application?
Optimizing the performance of a Spring Boot application involves various strategies. Utilizing caching mechanisms like Spring Cache can reduce database queries and enhance response times. Profiling the application using tools like Spring Boot Actuator helps identify performance bottlenecks. Implementing asynchronous programming using Spring's @Async annotation can improve concurrency and responsiveness.
Tips for Acing Spring Boot Interviews
Here are some invaluable tips for your Spring Boot interview strategies:
1. Research the Company: Before the interview, thoroughly research the company and its projects. Understand how Spring Boot fits into their tech stack and align your preparation accordingly.
2. Review Core Concepts: Ensure you have a strong grasp of the core Spring Boot concepts. Be prepared to explain the inversion of control, dependency injection, and Spring MVC's structure. Interviewers often assess your foundational knowledge, which forms the basis for more complex discussions.
3. Hands-on Projects: Undertake practical projects that involve Spring Boot. Building real-world applications showcases your ability to implement solutions and solve problems.
4. Code Walkthrough: Be ready to discuss the code you've written, especially if it's on your resume. Interviewers may ask about your thought process, design choices, and challenges faced during development. A clear explanation highlights your coding skills and decision-making abilities.
5. Problem-Solving: Technical interviews often include problem-solving scenarios. Approach these challenges systematically. Break down problems into smaller steps, articulate your thought process, and communicate your approach before writing code.
6. Behavioral Questions: Prepare for behavioral questions that assess soft skills. Highlight instances where you collaborated in a team, resolved conflicts, or took leadership.
Conclusion
As we conclude our journey through the realm of Spring Boot interviews, it's evident that preparation and confidence are the keys to unlocking success. The world of software development continues to evolve, and Spring Boot remains a vital tool for creating robust and efficient applications. Your mastery of Spring Boot concepts and the ability to apply them in practical scenarios can set you apart in the competitive landscape. Embrace the challenge, practice relentlessly, and leverage your unique experiences to leave a lasting impression on interviewers.
FAQs
What is Spring Boot?
A framework called Spring Boot, which is developed on top of the Spring framework, is intended to make application development simpler. A major draw of Spring Boot is its integrated support for technologies like Spring Data and Spring Security.
How does Spring Boot handle Dependency Injection?
A fundamental idea of Spring Boot is Dependency Injection (DI), which permits loose coupling between components. The Inversion of Control (IoC) container provided by Spring Boot manages the external source from which objects obtain their dependencies.
Explain the concept of Spring MVC in Spring Boot.
A web application design pattern called Spring MVC makes development easier by specifying the Model, View, and Controller components. The structure and capabilities provided by Spring MVC in Spring Boot allow for the management of user input, application logic, and data flow.
How does Spring Boot ensure data persistence?
Spring Boot works with the Java Persistence API (JPA) and Hibernate to provide effective data persistence in relational databases. Developers use JPA annotations to specify entities and relationships, and Spring Boot's automated configuration streamlines database operations.
How can Spring Boot applications be secured using Spring Security?
The authentication and authorization services that Spring Security offers to Spring applications are simple to implement into Spring Boot projects. Developers can secure endpoints and specify access restrictions by including the spring-boot-starter-security dependency and using annotations like @EnableWebSecurity.