Keep ‘em Separated: Get Better Maintainability in Web Projects Using the Model-View-Controller
Dive into the art of maintaining web projects effectively. Discover best practices, tools, and strategies for superior maintainability, ensuring the success of your projects.
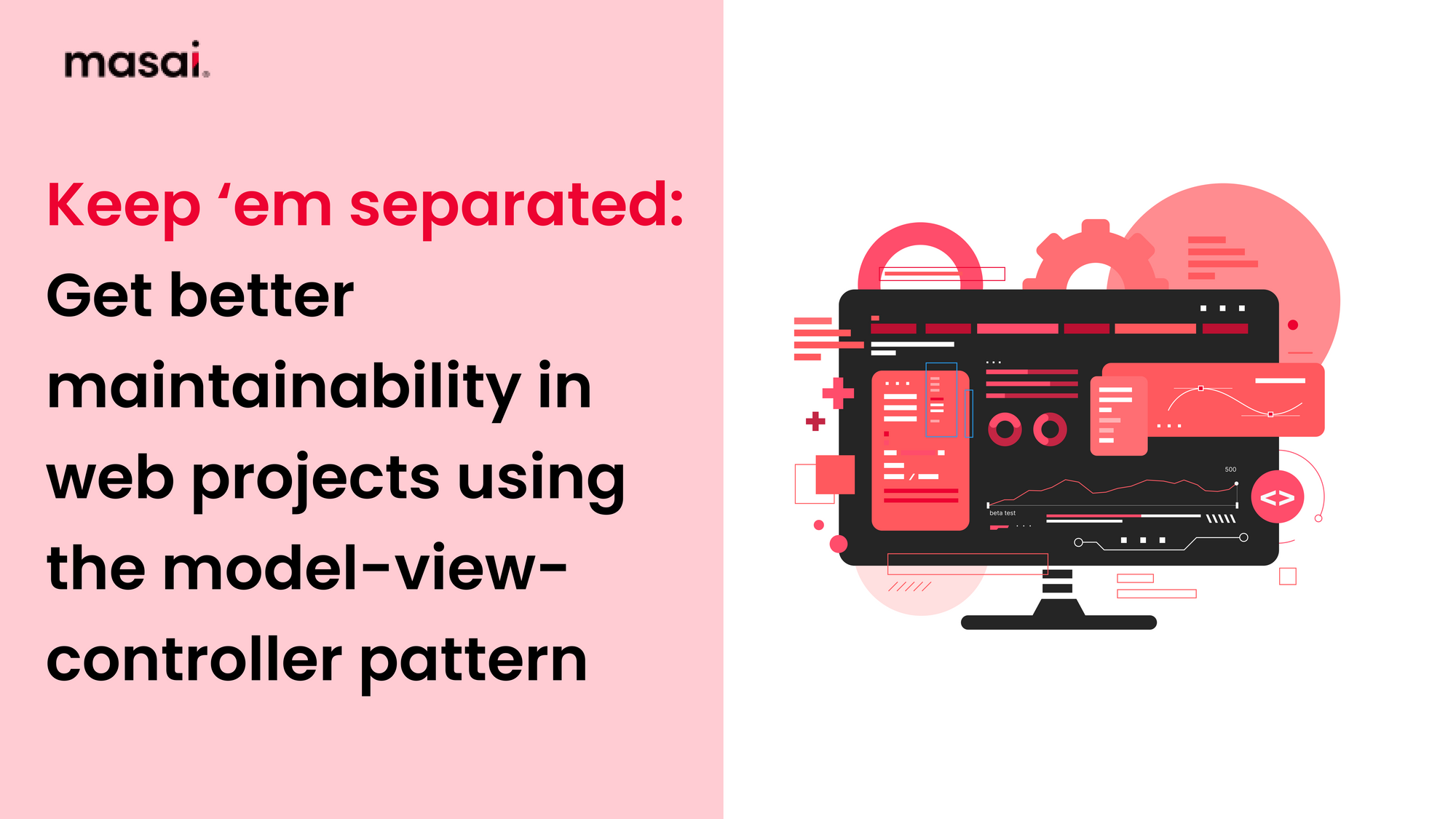
Introduction
In the fast-paced world of web development, building and maintaining complex web applications can be daunting. As your project grows in size and complexity, keeping your code organized, maintainable, and scalable becomes increasingly challenging. This is where architectural design patterns come into play, and one of the most popular and effective patterns for achieving maintainability in web projects is the Model-View-Controller (MVC) pattern.
This article will explore the MVC pattern in-depth, discussing its fundamental principles, benefits, and how to implement it in web development. By the end, you will clearly understand how MVC can help you keep your web projects organized, making them easier to develop, test, and maintain.
Understanding the MVC Pattern
The Model-View-Controller (MVC) pattern is a software architectural pattern that separates an application into three interconnected components: Model, View, and Controller. Each component has a specific responsibility, and they work together to provide a structured and maintainable way of developing web applications.
1. Model
The Model represents the application's core data and business logic. It is responsible for managing the data, processing it, and enforcing business rules. In a web application context, the Model typically interacts with a database, performing tasks like reading and writing data. It should be independent of the user interface and the presentation layer.
2. View
The View is responsible for presenting the data to the user. It displays the information retrieved from the Model in a user-friendly format. Views are often associated with HTML templates and user interface elements. Views should be passive in the MVC pattern and not contain business logic. Instead, they should rely on the Controller to interact with the Model.
3. Controller
The Controller acts as an intermediary between the Model and the View. It receives user input from the View and processes it, invoking the appropriate methods on the Model to perform the necessary actions. Controllers are responsible for handling user requests, managing the application flow, and updating the View with the latest data from the Model.
Benefits of Using MVC in Web Development
Now that we clearly understand the MVC pattern's components, let's explore its benefits when applied to web development.
1. Separation of Concerns
MVC enforces a clear separation of concerns within your web application. Each component has a distinct role and responsibility, making it easier to understand and maintain the codebase. Developers can work on individual components without affecting the others, which promotes code modularity and reusability.
2. Maintainability
One of the primary goals of the MVC pattern is to enhance maintainability. Organizing your code into separate components allows you to easily isolate and fix issues or add new features without risking unintended consequences. This modularity simplifies testing, as you can write focused unit tests for each component.
3. Scalability
As your web project grows, the MVC pattern allows you to scale it more efficiently. You can add or expand new features by creating new Models, Views, and Controllers as needed. The separation of concerns also makes it easier to onboard new developers, as they can focus on a specific component without an in-depth understanding of the entire application.
4. Reusability
MVC encourages code reusability by separating the presentation layer (View) from the business logic (Model). Views can be reused with different Controllers, and Models can be reused across various parts of your application or in entirely different projects. This reduces code duplication and promotes a more efficient development process.
5. Testing
Testing is an essential aspect of web development, and MVC facilitates it. Since each component has well-defined responsibilities, you can write comprehensive unit tests for Models, Controllers, and Views (to test their presentation logic). This leads to more robust and reliable code.
Implementing MVC in Web Development
Now that we've seen the advantages of using the MVC pattern, let's delve into how you can implement it in your web development projects.
Choose a Framework
Many web development frameworks provide built-in support for the MVC pattern. Depending on your programming language preference, you can choose from various options. For example:
Ruby on Rails: A popular MVC framework for Ruby.
Django: A high-level Python web framework that follows the MVC pattern.
Laravel: A PHP framework that also follows the MVC architectural pattern.
Selecting a framework that aligns with your language of choice can save you time and effort in setting up the basic structure of your project.
Design Your Database Schema (Model)
The first step in implementing MVC is designing your database schema. Your Model will interact with this database to perform CRUD (Create, Read, Update, Delete) operations. Ensure that your data schema is well-organized and follows best practices for data modeling.
Create Controllers
In MVC, Controllers handle the business logic of your application. They receive user requests, process them, and interact with the Model to retrieve or update data. When creating Controllers, keep them lean and focused on a single aspect of your application's functionality. This promotes code reusability and maintainability.
Develop Views
Views are responsible for presenting data to users. In web development, this often involves creating HTML templates and integrating them with your chosen framework's view engine. Views should be kept as simple as possible, containing minimal logic. Any dynamic data should be retrieved from the Model via the Controller.
Implement Routing
Routing is a crucial part of MVC. It defines how incoming requests are mapped to specific Controllers and actions. Your chosen framework will provide mechanisms for defining routes, typically in a configuration file. Ensure that your routing is logical and follows a consistent pattern.
Test Your Application
Testing is an integral part of the MVC development process. Write unit tests for your Models and Controllers to ensure they perform their intended functions correctly. Additionally, consider writing integration tests to validate the interaction between Controllers and Views.
Refine and Maintain
MVC is not a one-time setup but an ongoing process. As your application evolves, you may need to add new Controllers, Views, or Models. Ensure that you maintain the separation of concerns and keep your codebase clean and organized.
Real-World Examples of MVC in Action
To illustrate the practical application of the MVC pattern, let's consider two real-world scenarios: a simple to-do list application and a more complex e-commerce platform.
1. To-Do List Application
In a to-do list application, the MVC pattern can be implemented as follows:
Model: Manages tasks, stores them in a database, and provides methods for CRUD operations on tasks.
View: Displays tasks in a user-friendly format, allows users to mark tasks as complete and provides a form for adding new tasks.
Controller: Handles user requests, processes task-related actions (e.g., creating, updating, or deleting tasks), and interacts with the Model to retrieve or update task data.
2. E-Commerce Platform
For a more complex scenario like an e-commerce platform, the MVC pattern can be applied in the following way:
Model: Manages products, user accounts, orders, and payments. It interacts with the database to store and retrieve data and enforces business rules (e.g., calculating the total price of an order).
View: Presents product listings, shopping cart contents, and order confirmation screens. It also allows users to navigate product categories and place orders.
Controller: Handles user actions, such as adding products to the cart, processing orders, and managing user accounts. It interacts with the Model to perform these actions and updates the View accordingly.
In both examples, the MVC pattern promotes a clear separation of concerns. The Model handles data and business logic, the View focuses on presentation, and the Controller manages user interactions and application flow.
Common Misconceptions About MVC
While the MVC pattern is widely used and well-established, there are some common misconceptions that developers may encounter:
1. MVC Is Only for Large Projects
It's a misconception that MVC is only beneficial for large-scale applications. Even in smaller projects, MVC can help maintain code organization and make adding or modifying features easier. It provides a structured approach to development, regardless of project size.
2. MVC Is Limited to Web Development
While MVC is commonly associated with web development, it's not limited to this domain. The principles of MVC can be applied to various software applications, including desktop applications and mobile apps. The key is to separate concerns and achieve maintainability.
3. MVC Guarantees Perfect Separation
MVC provides a framework for achieving separation of concerns but doesn't guarantee a perfect separation in practice. Developers must still exercise discipline and adhere to best practices to maintain clean code and separation.
Conclusion
The Model-View-Controller (MVC) pattern is a powerful tool for achieving better maintainability in web development projects. By separating your application into three distinct components—the Model, View, and Controller—you can reap the benefits of improved code organization, maintainability, scalability, reusability, and testability.
Whether you're building a simple to-do list application or a complex e-commerce platform, MVC provides a clear and structured approach to development that can help you deliver robust and maintainable web applications. Embracing MVC as a fundamental design pattern in your web development toolkit will enable you to confidently and easily tackle projects of varying sizes and complexities.
FAQs
What is the MVC pattern in web development?
A web application is divided into three parts by the Model-View-Controller (MVC) pattern, a software architectural design. These parts are the Model, View, and Controller. The Controller controls user interactions and application flow, the Model handles data management and business logic, the View handles presentation.
How does MVC improve the maintainability of web projects?
Code separation is encouraged by MVC, which makes it simpler to manage, test, and maintain. Because each component has a distinct purpose, developers can work on one portion without influencing the others. This modularity makes updating, debugging, and adding new features easier.
Is MVC only suitable for large web projects?
No, MVC is advantageous for all sizes of projects. Although it's frequently linked with bigger systems, its advantages for maintainability extend to even smaller projects. Regardless of the size of the project, it offers an organized approach to development.
Can the MVC pattern be used in domains beyond web development?
MVC principles can be used in various software domains, including desktop and mobile applications. The essential idea is to separate issues to produce structured, maintainable code.
How do I implement the MVC pattern in my web project?
Select a framework that supports the MVC design pattern to implement it (such as Ruby on Rails, Django, or Laravel). Create a model for your database, write controllers to handle business logic, build views for presentation, and configure routing. A good MVC implementation depends on testing and ongoing maintenance.