Understanding Java Identifiers: Naming Conventions and Best Practices
Java identifiers are essential to Java programming because they let developers give each entity in their program a special name, preventing naming conflicts and enhancing the readability and maintainability of the code. Learn them in detail in this blog.
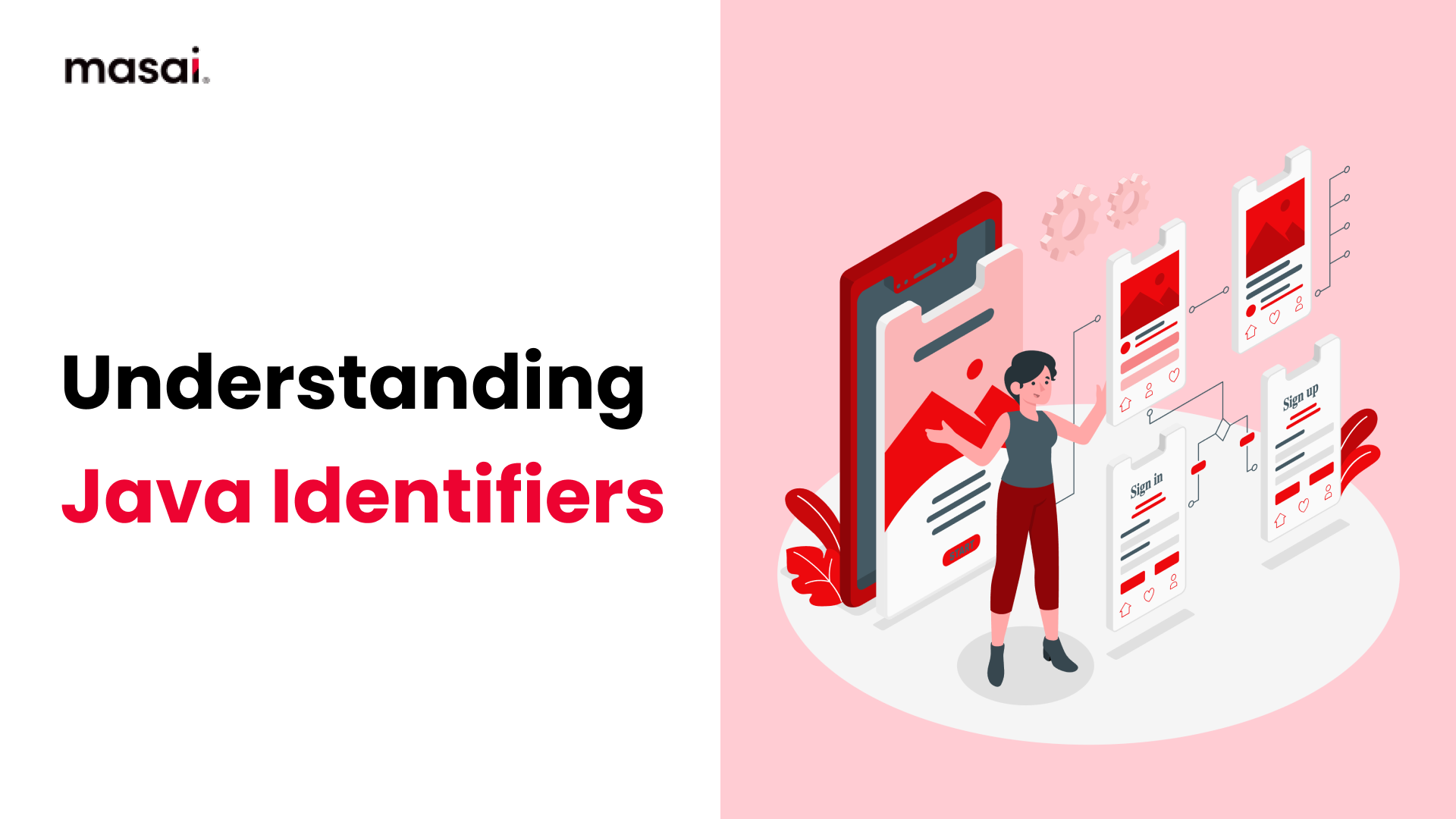
Introduction
Java programming involves creating and managing various variables, classes, methods, and packages. Giving them meaningful and consistent names is crucial for code readability, collaboration, and maintainability. In this blog, we'll understand what are Java identifiers, exploring naming conventions and best practices that empower developers to write clean and efficient code.
The Significance of Java Identifiers
Java identifiers are names that developers assign to different program elements to make them understandable. These elements encompass variables that store data, methods that perform actions, classes that define objects, and packages that organize code. By adhering to specific naming conventions and best practices, programmers ensure their code is understandable and conducive to teamwork.
Java Naming Conventions: Guidelines for Readable and Organized Code
Following consistent naming conventions in programming is like speaking a common language that computers and other programmers understand. These guidelines are a foundation for assigning names to different components within your Java code, encompassing variables, methods, classes, and constants. By following these principles, you not only enhance the readability of your code but also bolster the overall maintainability and collaborative dynamics within a development team. Let's explore these conventions in detail with examples for each type of identifier:
1. CamelCase for Classes and Interfaces When naming classes and interfaces, the CamelCase convention comes into play. Here's how it works:
- Start with a capital letter.
- Use capital letters for each new word.
- Do not use any spaces or underscores between words.
Example:
// Poor Naming
class studentprofile { ... }
// Following CamelCase
class StudentProfile { ... }
The first version lacks clarity in the example due to lowercase letters and no distinction between words. The improved version follows CamelCase, making the name more legible and descriptive.
2. camelCase for Methods and Variables For methods and variables, the convention shifts to camelCase, which maintains readability while distinguishing between words:
- Begin with a lowercase letter.
- Capitalize the first letter of each subsequent word.
Example:
// Poor Naming
int totalamount = calculateTotalAmount();
// Following camelCase
int totalAmount = calculateTotalAmount();
The initial version uses all lowercase letters, making the name hard to parse. Using camelCase, the revised version highlights individual words and clarifies the method's purpose.
3. UPPERCASE for Constants Constants, which represent values that remain unchanged, have their convention:
- Use all uppercase letters.
- Separate words with underscores.
Example:
// Poor Naming
final double pi = 3.14159;
// Following UPPERCASE with Underscores
final double PI = 3.14159;
In the first instance, the constant is named using lowercase letters, making it less noticeable. The second version adheres to the convention, making the constant easily distinguishable and recognizable.
4. Avoid Special Characters and Reserved Keywords While naming, avoiding special characters like @ or $ is essential. Also, refrain from using words that Java reserves for specific purposes, such as keywords like public, class, or static.
Example:
// Poor Naming (using reserved keyword)
int class = 10;
// Following the Convention
int numberOfClasses = 10;
Initially, a reserved keyword is used as an identifier, causing confusion and potential errors. By choosing a descriptive identifier like numberOfClasses, the code becomes more self-explanatory.
Best Practices for Choosing Identifiers
Creating effective identifiers goes beyond following conventions; it involves crafting names that enhance code clarity and maintainability:
- Descriptive Names: Choose names that convey the purpose of the element. For instance, instead of x, use numberOfStudents.
- Avoid Abbreviations and Acronyms: While abbreviations can save typing, overly cryptic names can hinder understanding. Opt for clarity over brevity.
- Use Nouns for Classes and Verbs for Methods: This helps convey the role of a class or method in the program's context.
- Singular vs. Plural: Choose singular or plural forms depending on whether the identifier represents one or multiple instances.
Benefits of Following Naming Rules and Enforcing Practices
- Enhanced Code Readability and Comprehension: Clear and consistent naming conventions lead to easy-to-read and understandable code. When variable names, method names, and class names follow a predictable pattern, it becomes effortless to identify the purpose and functionality of each element. This is akin to well-structured paragraphs in a book, where the words flow logically and make the content accessible to readers.
- Smooth Collaboration Among Team Members: Maintaining a uniform style and naming convention is crucial when multiple programmers collaborate on a project. Conventions ensure that regardless of who wrote a particular code, others can readily grasp its meaning. This harmony minimizes misunderstandings and fosters seamless collaboration. It's like speaking a common language everyone understands, making communication smoother and more effective.
- Reduced Maintenance Effort as Code Becomes Self-Documenting: Code that follows proper naming conventions becomes self-documenting. Instead of relying solely on comments to explain what each piece of code does, the names convey essential information. This eases the burden of maintaining the codebase over time.
Just as a well-labeled filing system eliminates the need to search for documents, self-descriptive code reduces the time spent deciphering functionality during updates or debugging.
- Enforcing Naming Conventions with Code Editors and IDEs: Modern code editors and Integrated Development Environments (IDEs) actively contribute to the adherence to naming conventions. They offer auto-suggestions as you type, helping you choose compliant names.
Furthermore, these tools often highlight non-compliant identifiers, drawing your attention to potential naming errors before they become a part of your codebase. It's akin to having a helpful assistant who ensures your writing follows grammar rules.
Conclusion
In Java programming, meaningful and consistent identifiers are vital in creating high-quality code. Developers create a path to efficient collaboration, improved code maintenance, and enhanced software quality by embracing naming conventions and adhering to best practices. The focus isn't solely on writing code; it encompasses crafting comprehensible, shareable, and adaptable code.
FAQs
Why do Java identifiers need naming conventions?
Naming conventions ensure uniformity in naming variables, methods, classes, and more, enhancing code readability and promoting consistent coding practices.
What is the significance of CamelCase and camelCase in Java?
CamelCase (e.g., StudentProfile) and camelCase (e.g., calculateTotalAmount) are naming styles. CamelCase starts with capital letters for each new word, while camelCase uses lowercase for the first word. These styles improve identifier clarity.
Why should constants be named in UPPERCASE with underscores?
Constants in UPPERCASE_WITH_UNDERSCORES (e.g., MAX_LENGTH) make them stand out, clearly indicating their unchanging nature and distinguishing them from variables.
How do naming conventions facilitate collaboration among programmers?
Uniform naming conventions establish a common language, making code more understandable and collaborative among developers. Consistency minimizes confusion and promotes smoother teamwork.
How do code editors and IDEs assist in enforcing naming conventions?
Code editors and IDEs offer auto-suggestions for compliant names and highlight non-compliant ones. These features aid developers in following conventions, catching errors, and maintaining naming consistency.