Acing the ReactJS Interview: Common Questions and Sample Answers
Prepare for your ReactJS interview with our detailed Q&A. Understand Virtual DOM, component lifecycle, state management, and more for interview success.
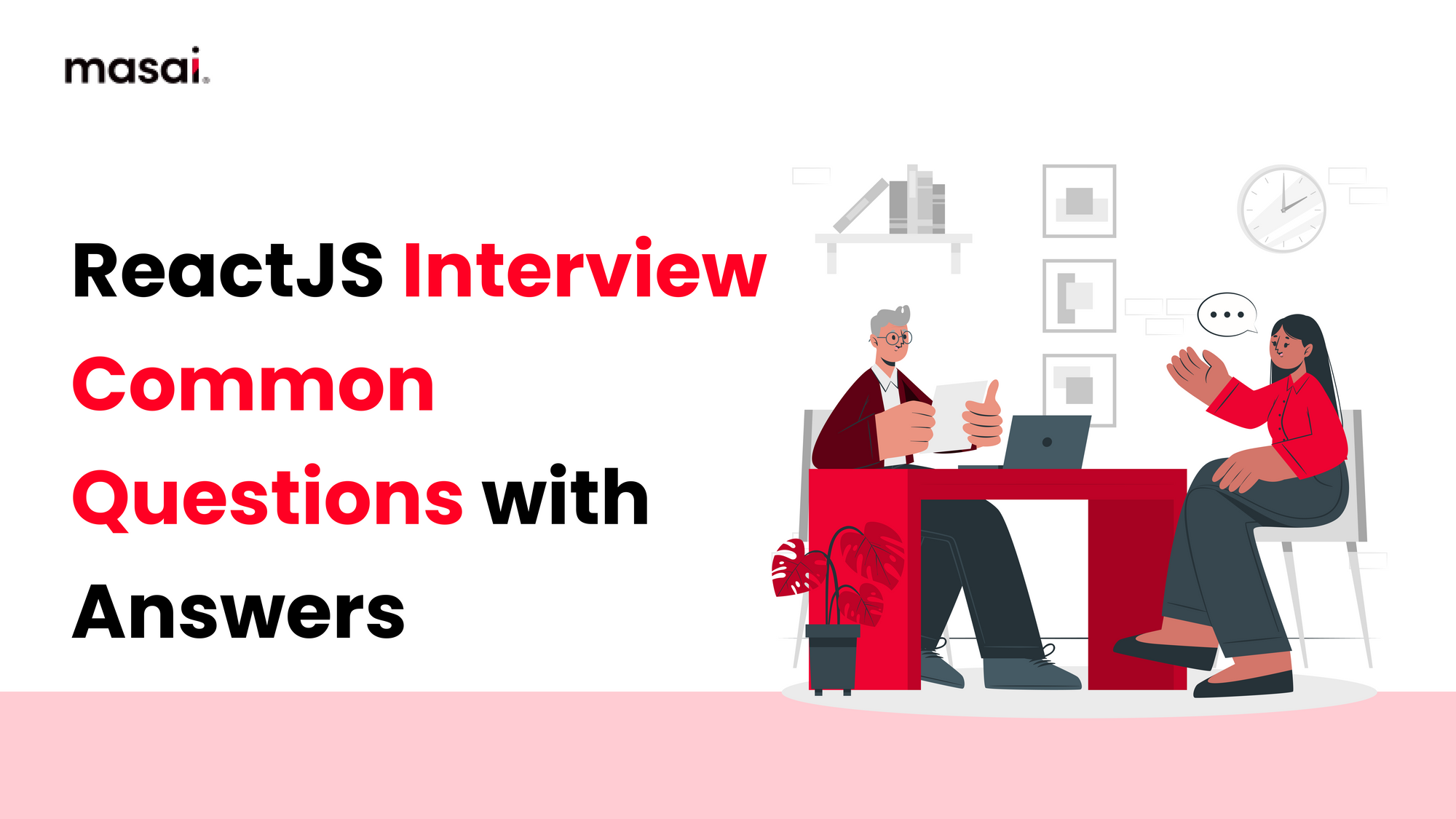
Introduction
In the dynamic landscape of modern web development, ReactJS has emerged as a cornerstone technology, revolutionizing how user interfaces are built and maintained. As React gains prominence, so does the demand for skilled ReactJS developers who can harness its power to craft seamless, interactive web applications. The ReactJS interview process is a pivotal gateway to these opportunities, testing candidates on their theoretical knowledge, coding prowess, and problem-solving acumen. This blog is your ultimate guide to facing and acing your ReactJS interview.
Common ReactJS Interview Questions and Sample Answers
Here are some common and frequently asked ReactJS interview questions and their answers.
1. What is ReactJS, and how does it differ from other JavaScript frameworks?
ReactJS is an open-source JavaScript library designed for building user interfaces. Unlike traditional frameworks, React focuses solely on the view layer, making it highly efficient for creating dynamic UIs. Its component-based architecture promotes reusability and modularity, allowing developers to compose complex interfaces from smaller, self-contained building blocks. This contrasts with frameworks like Angular, which offer a more comprehensive structure encompassing data management and routing. Additionally, React's virtual DOM optimizes rendering, updating only the necessary parts of the actual DOM, which enhances performance compared to other frameworks that might re-render entire sections.
2. Explain the concept of JSX.
JSX, or JavaScript XML, is a syntax extension used in React to describe the structure of UI components. It resembles HTML but seamlessly integrates JavaScript expressions. JSX simplifies component rendering by allowing developers to write expressive, declarative code. Under the hood, JSX gets transpiled into standard JavaScript using tools like Babel. For instance, <Button color="blue" /> in JSX corresponds to React.createElement(Button, {color: 'blue'}) in JavaScript. This blend of HTML-like syntax with JavaScript logic streamlines UI development and enhances code readability.
3. What is the significance of the virtual DOM?
Virtual DOM is a crucial concept in React that boosts performance and efficiency. It's an abstraction of the actual DOM, a lightweight representation of UI components and their structure. When changes occur, React compares the virtual DOM with the real DOM, computing the minimal necessary updates. This leads to fewer direct manipulations of the actual DOM, reducing rendering overhead and enhancing speed. Consequently, applications built with React deliver a smoother user experience as updates are processed optimally, minimizing unnecessary reflows and repaints.
4. How do React props work?
React props, short for properties, pass data from a parent component to its child components. They enable communication and allow components to be reusable and modular. Props are immutable and provide a way to share information between components without directly modifying the component's state. By defining attributes within JSX tags, you can pass data down the component hierarchy. For instance, <UserProfile name="John" age={25} /> sends the name and age values as props to the UserProfile component. Inside the component, these values are accessible as this.props.name and this.props.age.
5. What is React state, and how is it different from props?
React state is a built-in feature that allows components to store and manage their internal data. While similar to props, which are passed from parent to child components, the state is local and mutable, whereas props are immutable. The state is primarily used for dynamic data, like user input or component-specific values, that can change over time. Unlike props, which are externally controlled, a component manages its state. Using the setState method, you can modify the state, prompting React to re-render the component and update the UI based on the changes.
6. Explain the component lifecycle in React.
The React component lifecycle consists of three main phases: mounting, updating, and unmounting. The component is initialized during mounting, and methods like constructor and componentDidMount are executed. In the updating phase, changes trigger methods like shouldComponentUpdate to determine if re-rendering is needed, followed by componentDidUpdate after rendering. Finally, in the unmounting phase, the component is removed from the DOM, and the component will unmount method is called. Understanding these phases helps manage component behavior, optimize rendering, and perform cleanup tasks.
7. How does React handle forms?
React provides two approaches for handling forms: controlled components and uncontrolled components. Controlled components maintain form data in the component's state, enabling dynamic updates and validation. Uncontrolled components, on the other hand, rely on the DOM for form data storage. Developers can access form values using references after user input. Controlled components offer better control and validation, making them a preferred choice for complex forms, while uncontrolled components are suitable for simpler scenarios.
8. What is the significance of keys in React lists?
Keys are essential when rendering lists of elements in React. They provide a unique identifier for each item in a list, enabling React to track and manage updates efficiently. When items are added, removed, or rearranged, keys ensure that only the necessary elements are modified in the DOM. React might re-render the entire list without keys, resulting in performance issues and unintended side effects. Assigning a key to each list item ensures stable and predictable rendering behavior.
9. How can you optimize a React application's performance?
Optimizing a React application's performance involves techniques like code splitting, lazy loading, and memoization. Code splitting divides the application into smaller chunks, loaded on-demand, reducing the initial load time. Lazy loading defers loading non-essential components until they are needed. Memoization optimizes rendering by caching the results of expensive function calls using React. memo and useMemo, preventing unnecessary recalculations.
10. Describe the concept of "lifting state."
"Lifting state up" is a pattern in React where the shared state is managed at a higher level in the component hierarchy and passed down as props to child components. This pattern enhances data consistency and synchronization between components. By centralizing state management, you prevent inconsistencies and improve maintainability. For instance, when multiple child components need access to the same data, lifting the state to a common ancestor simplifies data management, reduces redundancy, and enhances component interaction.
Conclusion
In the ever-evolving realm of web development, ReactJS is a beacon of innovation, shaping the digital landscape with its dynamic capabilities. As you embark on your ReactJS interview journey, remember that success goes beyond mastering technical concepts – it encompasses effective communication and the ability to showcase your skills with clarity. By embracing the insights shared in this guide, you can navigate the diverse ReactJS interview questions confidently. Your journey to ReactJS excellence starts here. Best of luck!
FAQs
What should I anticipate from a ReactJS job interview?
Your theoretical knowledge, technical skills, and problem-solving talents are evaluated during ReactJS interviews. React principles, JSX, virtual DOM, state management, and component lifecycle are topics that interviewers frequently probe.
What distinguishes ReactJS from other JavaScript frameworks?
A JavaScript library devoted to creating user interfaces is called ReactJS. It excels at building dynamic user interfaces by quickly drawing just the required bits of the view. React's component-based architecture, in contrast, to complete frameworks like Angular, encourages reusability and modularity, making it a popular option for front-end development.
Can you describe JSX and how it is used to create React?
React uses the grammar extension known as JSX, or JavaScript XML, to express UI components. It simplifies component rendering and improves code readability by combining JavaScript expressions with syntax similar to HTML. The declarative nature of React programming is enhanced by translating JSX into regular JavaScript code.
In React, what role does the virtual DOM play?
React's virtual DOM is a key idea that improves performance. It optimizes UI rendering by computing the fewest updates possible and is a lightweight approximation of the actual DOM.
How are props and React states different?
React state is mutable and used to manage internal data within components. Props, on the other hand, are immutable and handed from parent to child components. Props enable communication between components without directly affecting their internal state, whereas the state is appropriate for maintaining dynamic data that might change over time