Mastering Java Variables: Declaration, Scope, and Usage
One of the key concepts in any programming language is the concept of variables. This in-depth article will explore the world of Java variables, including their types, syntax, scope, and best practices for utilizing all of their capabilities.
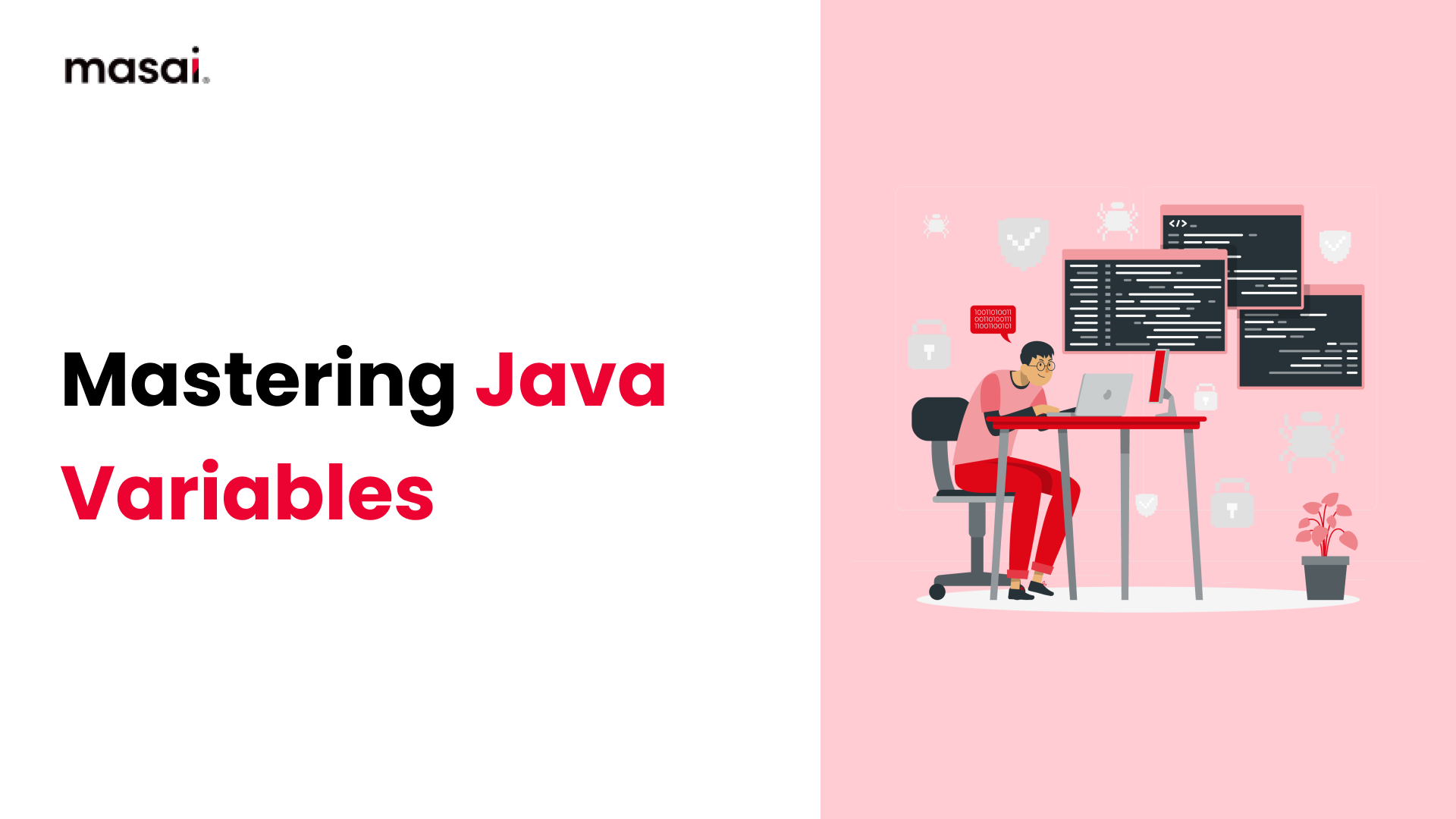
Introduction
Java, a powerful programming language known for its strength and flexibility, is widely used to create various types of software. It's everywhere, from computer programs to mobile apps and online systems. At the heart of Java programming are variables – these are like dynamic tools that help store and work with data. In fact, variables are absolutely essential in any Java program. In this simple guide, you'll uncover the basics of Java variables. Discover their declaration process, understand their scope, and explore the various methods they're put to use.
Declaration of Variables
In Java programming, determining variables is essential before their utilization becomes feasible. This declaration process encompasses the specification of the variable's data type, the allocation of a suitable name, and the potential assignment of an initial value. The basic syntax for variable declaration is as follows:
data_type variableName = initial_value;
Here's a breakdown of the components:
- data_type: This represents the type of data the variable can hold. Java has various predefined data types, encompassing integers, floating-point numbers, boolean values, single characters, etc.
- variableName: This is the identifier you give to the variable. Following Java's naming conventions is imperative, which includes commencing with a letter, abstaining from spaces, and utilizing camelCase for names spanning multiple words.
- initial_value: This is an optional step to assign an initial value to the variable. Without value assignment during declaration, a variable adopts a default value contingent upon its data type. For instance, integers default to 0, floating-point numbers to 0.0, booleans to false, characters to '\u0000', and reference types to null.
Consider the following instance of variable declaration:
int age = 25;
double salary = 50000.0;
boolean isStudent = true;
char initial = 'J';
String name = "John";
Scope of Variables
The variable's scope pertains to the section of the code wherein the variable can be reached and utilized. Varied levels of visibility are attributed to Java variables, dictating the locations they can be retrieved from. In Java programming, three primary levels of variable scope exist:
1. Local Variables: These variables are declared within a code block, such as a method, constructor, or loop. They are only accessible within that block and its nested blocks. Before utilizing local variables, it is necessary to initialize them. The memory assigned to these local variables is released upon exiting the code block. This promotes memory efficiency.
2. Instance Variables (Non-Static Variables): These variables are declared within a class but outside any method, constructor, or block. They belong to an instance of the class and can have different values for different instances. Instance variables are created when an object of the class is instantiated and are accessible as long as the object exists.
3. Class Variables (Static Variables): These Variables are declared within a class but possess a static designation. These variables are shared among all class instances, effectively transforming into attributes of the entire class instead of individual objects. Upon the class being loaded, these variables get initialized and endure in memory throughout the program's runtime."
To demonstrate variable scope, let's examine the subsequent illustration:
public class ScopeExample
{
int instanceVar = 10; // This represents an instance variable.
public void methodA()
{
int localVar = 5; // This denotes a local variable confined to methodA.
// Displaying instanceVar accessible here:
System.out.println(instanceVar);
// Displaying localVar accessible here:
System.out.println(localVar);
}
public static void main(String[] args)
{
ScopeExample obj = new ScopeExample();
obj.methodA();
// The next line would lead to an error since localVar isn't reachable here:
// System.out.println(localVar);
}
}
In this scenario, the instanceVar remains accessible in both the instance and main methods. However, the localVar exclusively retains accessibility within the methodA. Any attempt to access localVar within the main method would culminate in a compilation error.
Usage of Variables
Serving as containers for data that can be manipulated and utilized in diverse ways, variables in Java play a crucial role. Java variables find application in various scenarios, including:
1. Data Storage: Storing data, like numbers, text, and other information types, within variables facilitates their use in calculations, processing, and display.
2. Control Flow: Employing variables in Java enables the regulation of program flow through conditional statements (such as if and switch) and loops (including for and while).
3. Manipulation: Variables undergo processes like mathematical operations, string concatenation, and other transformations, resulting in attaining desired outcomes.
4. Object Interaction: Variables can store object references, allowing interaction with complex data structures and methods defined within objects.
5. Method Parameters: Variables can be passed as parameters to methods, allowing them to be used and modified within the method's scope.
6. Scope Isolation: Local variables provide scope isolation, preventing unintended interference between different parts of the code.
7. Global Data: Instance and class variables hold data that needs to be accessible across different methods or objects in a class.
Conclusion
In programming languages, variables serve as fundamental components, a truth that also holds for Java. It is crucial to grasp the art of declaring, overseeing scope, and employing variables efficiently, a skill that forms the foundation of any software developer course. Mastering the principles expounded upon in this blog post will give you ample capability to wield Java variables to their fullest potential, enabling the crafting of resilient and optimal applications.
FAQs
What is the purpose of declaring variables in Java?
Variables in Java serve as placeholders to store and manage data during program execution. They allow you to manipulate values, control program flow, and interact with different parts of your code.
How do I choose appropriate variable names in Java?
When naming variables in Java, follow these guidelines:
• Use descriptive names that convey the purpose of the variable.
• Start with a letter and use camelCase for multi-word names (e.g., studentAge, totalAmount).
• Avoid using reserved keywords and special characters in names.
What is the difference between instance variables and local variables?
• Instance variables: These are declared within a class but outside methods. They are associated with instances (objects) of the class and have different values for each instance.
• Local variables: These are declared within methods or blocks and are only accessible within their scope. They must be initialized before use and are used for temporary storage.
Can a final variable's value be modified in Java?
Modifying the value of a variable marked as final after the assignment is not allowed. A final variable's value remains constant throughout its lifespan, effectively representing a constant.
What does the scope of a class variable (static variable) encompass in Java?
Class variables (static variables), belonging to the class itself rather than specific objects, are shared among all class instances. Possessing a scope at the class level, these variables can be accessed within all methods and blocks of the class. Initialization of class variables occurs upon class loading, and they persist in memory throughout the program's runtime.