Preparing for Java Interviews: Common Questions and Tips
To improve your chances of succeeding in the interviews, go over all the questions. The questions are going to focus on the principles of Java at the basic, core, and advanced levels. So let's study a range of helpful Java Technical Interview Questions and Answers in depth.
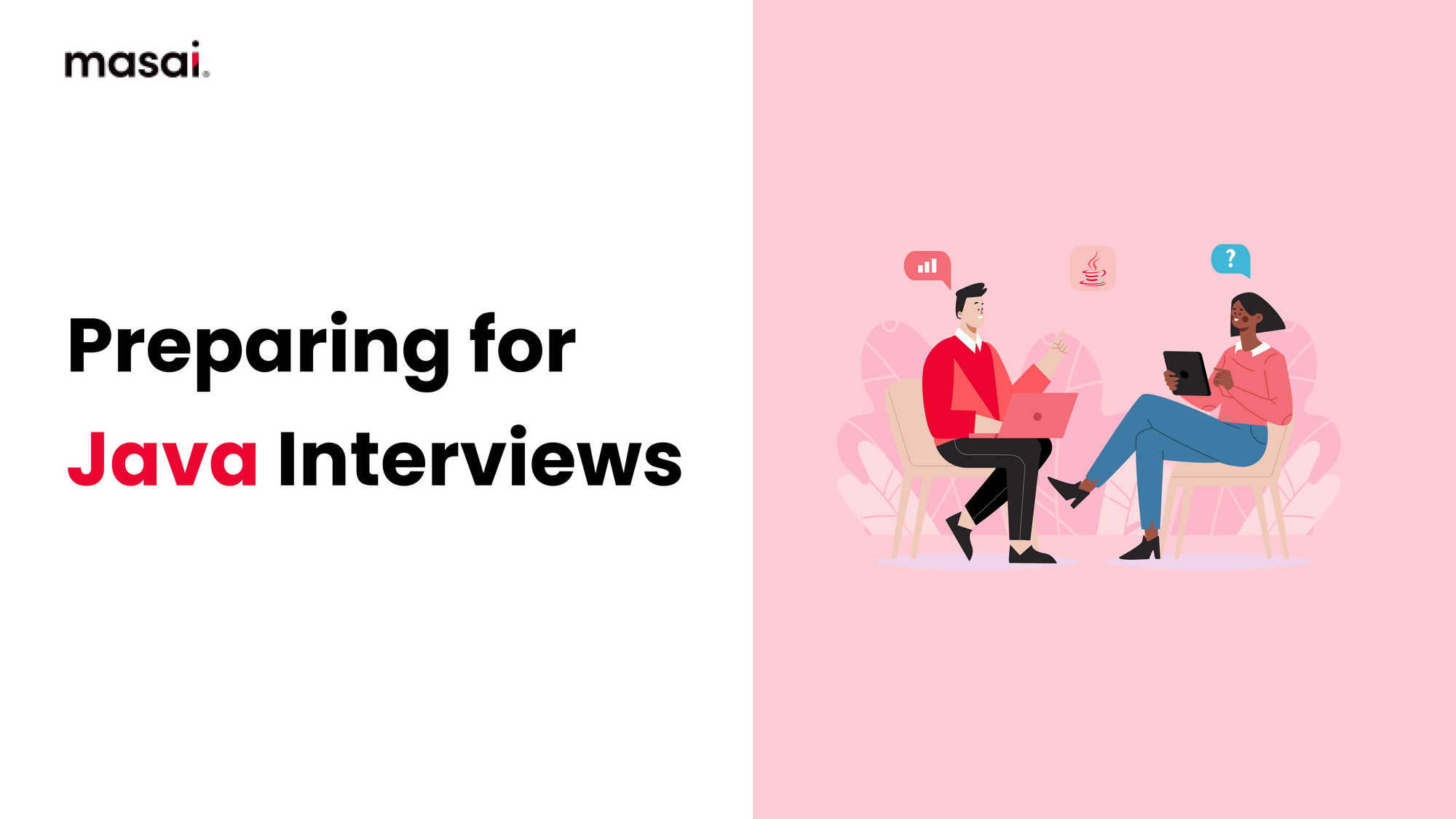
Introduction
Among the programming languages, Java stands strong as a high-level favorite. It emerged with a focus on simplicity and cross-platform compatibility. Java's ubiquity extends to mobile devices and laptops, sustaining demand for skilled Java developers.
Whether your sights are set on game or Android development, mastering Java could steer you toward a fulfilling web developer career. Java's vastness includes diverse concepts that interviewers might explore. Freshers could tackle fundamentals like Strings, Collections, and OOPs, while experienced individuals could delve into more nuanced aspects. We provide a thorough guide to acing Java interviews that includes common questions and sample answers, as well as tips to help you on your way.
Most Popular Interview Questions in Java
Here are some of the most frequently asked and common Java interview questions and their answers.
Why is Java a platform-neutral language?
Answer: Java's platform independence is due to its byte codes. These codes can function on any computer, regardless of its underlying operating system. This attribute enables Java to operate uniformly across diverse systems.
Why isn't Java considered 100% Object-oriented?
Answer: Java isn't classified as completely object-oriented due to its utilization of eight primitive data types: boolean, byte, char, int, float, double, long, and short. These types are not treated as objects within the language.
What Are Wrapper Classes in Java?
Answer: Wrapper classes in Java are intermediaries between primitive data types and reference types (objects). For each primitive data type, there's a dedicated class. They earn their name as "wrapper" classes because they encapsulate primitive data within an object of their respective class.
Does Java allow classes to have multiple inheritances?
Answer: No, Java doesn't permit multiple inheritances for classes. The decision was driven by simplicity and to sidestep the "Deadly Diamond Problem." This problem arises when a child class inherits from two parent classes that provide conflicting methods for implementing a particular feature.
How does Java differ from C?
Answer: Java and C are object-oriented programming languages but exhibit distinct contrasts in various aspects.
1. Platform Independence: Java boasts platform independence, allowing its code to operate across platforms with a Java virtual machine (JVM). In contrast, C is platform-dependent, confined to the system it's compiled for.
2. Memory Management: Java employs automatic memory management, freeing programmers from manual memory allocation. C, on the other hand, necessitates manual memory management, requiring careful handling to prevent memory leaks.
3. Safety: Java prioritizes safety, integrating built-in security features and guarding applications against malicious code. It restricts direct access to the operating system, fortifying applications against security breaches.
4. Performance: While Java offers portability and security, C generally delivers faster performance. The abstraction layer introduced by Java's virtual machine can impact speed, whereas C excels in high-performance applications needing direct OS interaction.
List the Hibernate framework's core interfaces.
Answer: Here is a list of the core interfaces within the Hibernate framework:
- Configuration
- Session
- SessionFactory
- Criteria
- Transaction
- Query
Is Thread Safety Guaranteed for Singleton Beans?
Answer: Singleton beans do not inherently ensure thread safety. While singletons are a blueprint for creation, thread safety pertains to execution considerations.
Explain the difference between equals() and == in Java.
Answer: Understanding the Distinction between equals() and == in Java:
The equals() method, present in the Object class of Java, assesses the equality of two objects based on business logic specifications.
On the other hand, the "==" operator, known as the equality operator, is a binary operator in Java. It facilitates the comparison of both primitives and objects. The Object class offers a public boolean equals(Object o) method. By default, this method employs the == operator to compare two objects. This behavior can be modified, much like in the String class. Notably, the equals() method is harnessed to compare the actual values of two objects.
Why Java Avoids Pointers?
Answer: Java deliberately avoids pointers due to safety concerns and program complexity. Java's hallmark is its code simplicity, and introducing pointers would contradict this principle. Additionally, the Java Virtual Machine (JVM) handles memory allocation automatically, aiming to prevent direct memory access by users. This design discourages the adoption of pointers in Java, enhancing both security and ease of programming.
Explain the Java main() Method.
Answer: Java's main() method serves as the entry point for every Java program. It's consistently written as the public static void main(String[] args).
Public: "Public" is an access modifier, determining who can use this method. It signifies that this method is open for access by any class.
Static: This keyword signifies that main() is class-based. By making main() static, it can be accessed without creating an instance of a class. If main() isn't static, an error arises because main() is invoked by the JVM before objects exist, and only static methods can be directly called via the class.
Void: The return type of the method. A void indicates a method that doesn't produce any output.
Main: It's the method name the JVM seeks as the starting point for an application, following a specific signature. The main execution transpires here.
String args[]: This parameter is passed to the main method, allowing it to receive command-line arguments.
Crucial Interview Tips
Let's now look at a few essential tips for your road toward a job in coding.
1. Solid Fundamentals: Strengthen your core Java concepts, including object-oriented programming, data structures, algorithms, and design patterns.
2. Code Practice: Regularly practice coding on platforms like LeetCode, HackerRank, and CodeSignal to enhance problem-solving skills.
3. Resume Highlighting: Tailor your resume to emphasize relevant projects, technologies, and achievements. Be ready to explain your contributions in detail.
4. Algorithm Proficiency: Focus on standard interview algorithms, such as sorting, searching, and dynamic programming. Understand their time and space complexities.
5. Data Structures Mastery: Be well-versed in various data structures like arrays, linked lists, trees, graphs, and hash maps. Understand when and how to use each effectively.
6. System Design: Brush up on system design principles. Be prepared to discuss scalability, database design, API design, and trade-offs in real-world scenarios.
7. Behavioral Questions: Prepare for behavioral questions by practicing concise and relevant answers that showcase your teamwork, problem-solving, and communication skills.
8. Mock Interviews: Conduct mock interviews with peers or mentors to simulate real interview scenarios. This helps in building confidence and improving your performance.
9. Coding Patterns: Familiarize yourself with common coding patterns used in Java interviews, such as sliding windows, two-pointers, and backtracking.
10. Ask Questions: At the end of the discussion, ask thoughtful questions about the company's projects, team structure, and culture to demonstrate your genuine interest and engagement.
The Bottom Line
And with that, we conclude this blog. The subjects covered in these Core Java Interview Questions encompass the highly sought-after questions employers seek in Java Professionals. The interview questions and tips above can help you increase your chances of getting the best job in the software development domain. Success in interviews depends on a candidate's technical expertise, problem-solving abilities, and good communication. If you make the necessary preparations and have a positive mindset, you'll have a far better chance of succeeding in Java programming interviews.
FAQs
Why does Java avoid using pointers?
Java purposefully stays away from pointers owing to code complexity and safety issues. Pointers are not present because Java places a strong emphasis on security and simplicity. To improve security, the Java Virtual Machine (JVM) also manages memory allocation automatically.
What's the significance of the main() method in Java?
Every Java program's entry point is the main() function. The Java Virtual Machine (JVM) can launch the program's execution with the command "public static void main(String[] args)". Without generating instances, direct access is made possible using the "static" keyword.
What is the importance of system design knowledge in Java interviews?
Knowledge of system design is essential because it demonstrates your capacity to create scalable and effective solutions. To show off your knowledge, be prepared to talk about scalability, database design, API design, and trade-offs in practical contexts.
How can I excel in both technical and behavioral aspects of Java interviews?
By enhancing your technical expertise, problem-solving capabilities, and communication abilities, you can balance your preparedness. Prepare succinct responses that highlight your teamwork and communication skills while you practice coding, research algorithms, and analyze data structures. Perform practice interviews to improve your performance as a web developer.
How can I enhance my chances of success in Java interviews?
Develop your foundational skills, practice coding frequently, emphasize key skills on your CV, grasp common algorithms and data structures, and become familiar with system design ideas. To increase confidence, practice interviews and prepare for behavioral questions.