Enhancing Problem-Solving Skills in Java Programming: A Comprehensive Guide
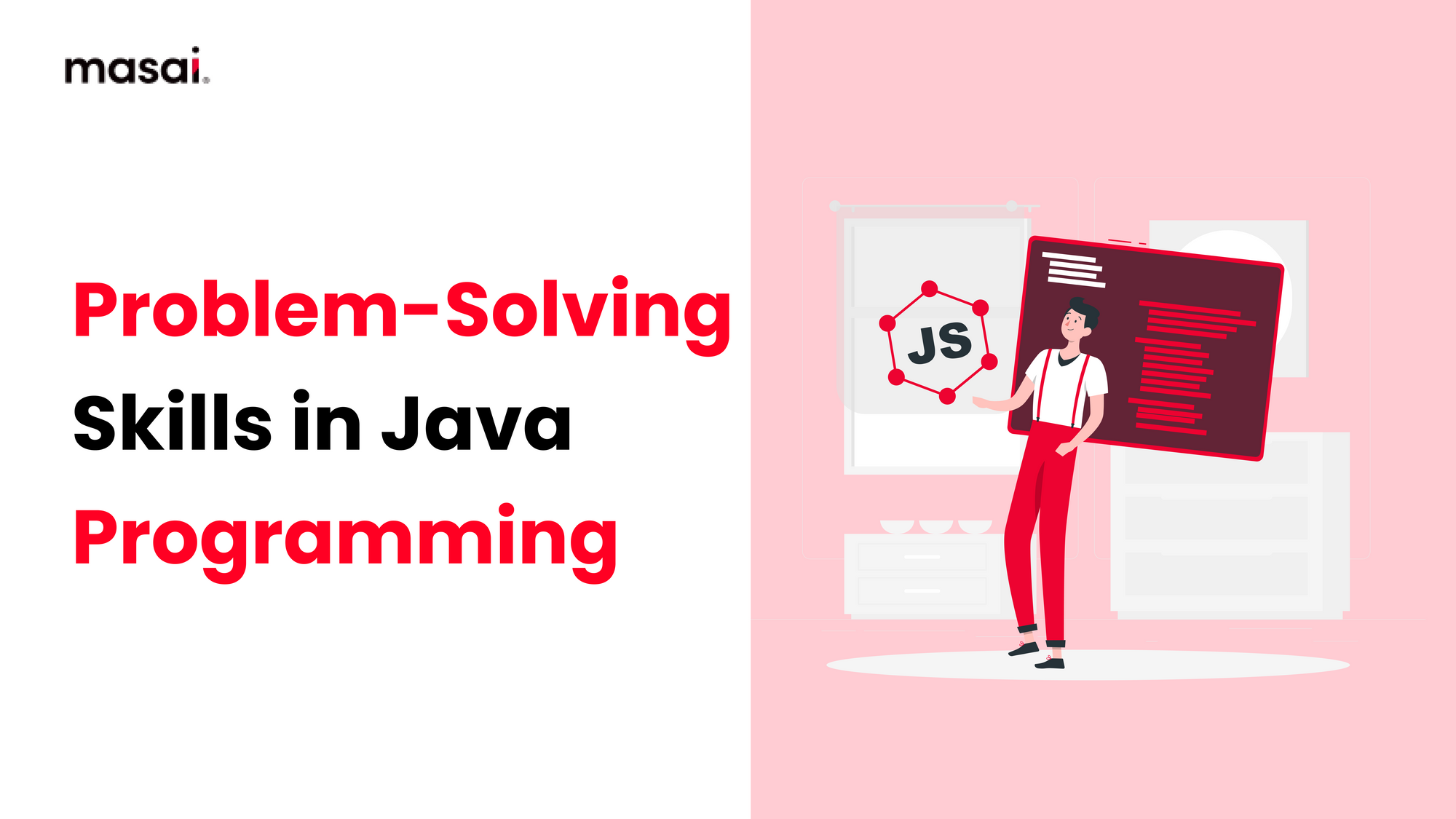
Introduction
Problem-solving skills lie at the heart of successful Java programming. In the realm of software development, the ability to dissect complex challenges, design efficient solutions, and implement them through code is paramount. This article delves into the art of honing problem-solving skills within the context of Java programming. By combining logical thinking, algorithmic strategies, and effective coding practice, developers can elevate their problem-solving skills in Java programming and create elegant, robust solutions.
Understanding Problem-Solving in Java Programming
Problem-solving in the realm of Java programming refers to the systematic approach of identifying, analyzing, and solving coding challenges. In a software developer course, you’ll learn that it involves breaking down intricate problems into manageable components and devising logical strategies to tackle them. Logical thinking and algorithmic understanding play a pivotal role in this process, allowing developers to construct well-structured and optimized solutions. Such skills are indispensable for crafting software that is not only functional but also efficient.
Guidelines for Enhancing Problem-Solving Skills
Here’s a rundown of the guidelines to enhance your problem-Solving skills.
- Breaking down complex problems: Start by breaking down a complex problem into smaller sub-problems. This approach simplifies the overall challenge and makes it easier to develop individual solutions for each component.
- Developing a systematic approach: Establish a systematic approach to problem-solving. Define the problem, identify the required inputs and desired outputs, and outline the steps needed to bridge the gap.
- Understanding before coding: Resist the urge to immediately dive into coding. Spend ample time understanding the problem statement, its nuances, and potential edge cases. A solid understanding is the foundation of an effective solution.
- Utilizing pseudocode and flowcharts: Before writing actual code, create pseudocode or flowcharts to outline the logical flow of your solution. This helps in visualizing the process and identifying potential flaws early on.
- Incremental development and testing: Build your solution incrementally, testing each component as you progress. This approach allows you to catch errors early and refine your solution iteratively.
Effective Coding Practice for Problem-Solving
Regular coding practice is essential for honing problem-solving skills. Engage in a variety of coding challenges that span different difficulty levels and problem domains. Online coding platforms, such as LeetCode, HackerRank, and CodeSignal, offer a wealth of practice problems and allow you to benchmark your skills against a global community of programmers. Mastering problem-solving skills in Java programming involves a combination of understanding the language's features, algorithms, and data structures, as well as practicing systematic approaches to tackling challenges.
Applying Algorithms for Efficient Solutions
Algorithms are step-by-step instructions for solving a specific problem. Learning and implementing various algorithms can greatly improve your problem-solving capabilities. Start by understanding the fundamental algorithms such as sorting, searching, and graph traversal. As you become more comfortable, move on to more advanced algorithms like dynamic programming, greedy algorithms, and divide-and-conquer techniques. Regularly practicing these algorithms through coding exercises will help you develop a toolkit of strategies to approach different types of problems effectively.
Strengthening Logical Thinking Abilities
Logical thinking is the foundation of problem-solving. It involves breaking down complex problems into smaller, manageable parts and understanding the relationships between different elements. To enhance your logical thinking skills, practice solving puzzles, brain teasers, and mathematical problems. Additionally, try to analyze the structure of various programming challenges and identify patterns and dependencies. Cultivating this skill will enable you to devise creative and efficient solutions to intricate programming problems.
Learning from Real-world Examples
Real-world examples provide practical insights into applying problem-solving skills in Java programming. Let's explore a few examples:
Example 1: Finding the factorial of a number: Break down the problem into smaller steps, creating a loop to multiply consecutive integers.
Example 2: Implementing binary search: Utilize the divide and conquer strategy to narrow down the search space and efficiently find the target element.
Example 3: Solving the Fibonacci sequence: Employ dynamic programming to optimize recursive calculations and generate Fibonacci numbers efficiently.
Overcoming Common Challenges
When solving programming problems, you're likely to encounter common challenges such as bugs, runtime errors, and inefficiencies. Embrace these challenges as opportunities to learn. Debugging is a crucial aspect of problem-solving. Cultivate a systematic approach to identifying and fixing errors by using tools like debugging environments and print statements. Additionally, optimize your code by analyzing time and space complexity, identifying bottlenecks, and making informed decisions to improve efficiency.
Putting Problem-Solving Skills to the Test
Practicing problem-solving skills is essential for improvement. Utilize coding platforms like LeetCode, HackerRank, and Codeforces to access a wide range of programming challenges. These platforms often categorize problems by difficulty, allowing you to gradually progress from easy to more complex tasks. Set aside dedicated time for consistent practice, and challenge yourself by attempting problems that initially seem daunting.
Collaborate with fellow programmers, engage in coding competitions, and participate in online forums to gain exposure to different problem-solving approaches.
Conclusion
An essential talent for Java programmers is the ability to solve problems. Developers can become skilled problem solvers by following logical rules, practicing code often, learning algorithms, and cultivating logical thought. The secret to maximizing the potential of problem-solving abilities is constant learning combined with practical experience. As you set out on this path, keep in mind that every obstacle you overcome will help you get closer to mastering Java programming and being able to create unique, effective solutions.
FAQs
How can I improve my problem-solving skills in Java programming?
Enhance your problem-solving skills in Java by practicing algorithmic challenges, breaking down complex problems into smaller tasks, and leveraging data structures effectively. Regular coding practice and participating in coding competitions can also contribute to your skill development.
What strategies can I employ to tackle challenging Java programming problems?
Approach difficult Java programming problems step by step, analyze the problem requirements thoroughly, devise a clear plan, and implement it incrementally. Utilize debugging tools and collaborate with peers to gain different perspectives on problem-solving techniques.
Are there any specific resources for practicing Java problem-solving?
Numerous online platforms offer Java programming challenges and exercises, such as LeetCode, HackerRank, and Codeforces. These resources provide a range of problems to solve, helping you refine your problem-solving skills and Java proficiency.
How do I optimize my Java code for better problem-solving outcomes?
Optimize Java code by choosing efficient data structures, minimizing unnecessary iterations, and employing dynamic programming or memoization techniques when appropriate. Regularly review and refactor your code to improve its clarity and performance.
Q. Can problem-solving in Java programming benefit my overall programming proficiency?
Enhancing your problem-solving skills in Java translates to improved programming proficiency in general. The analytical mindset and structured problem-solving techniques you develop will prove valuable across various programming languages and domains.