Cracking Data Structure and Algorithm Interviews: Common Questions
Unlock the secrets to acing data structure and algorithm interviews. Explore common questions, master algorithms, and shine in technical assessments.
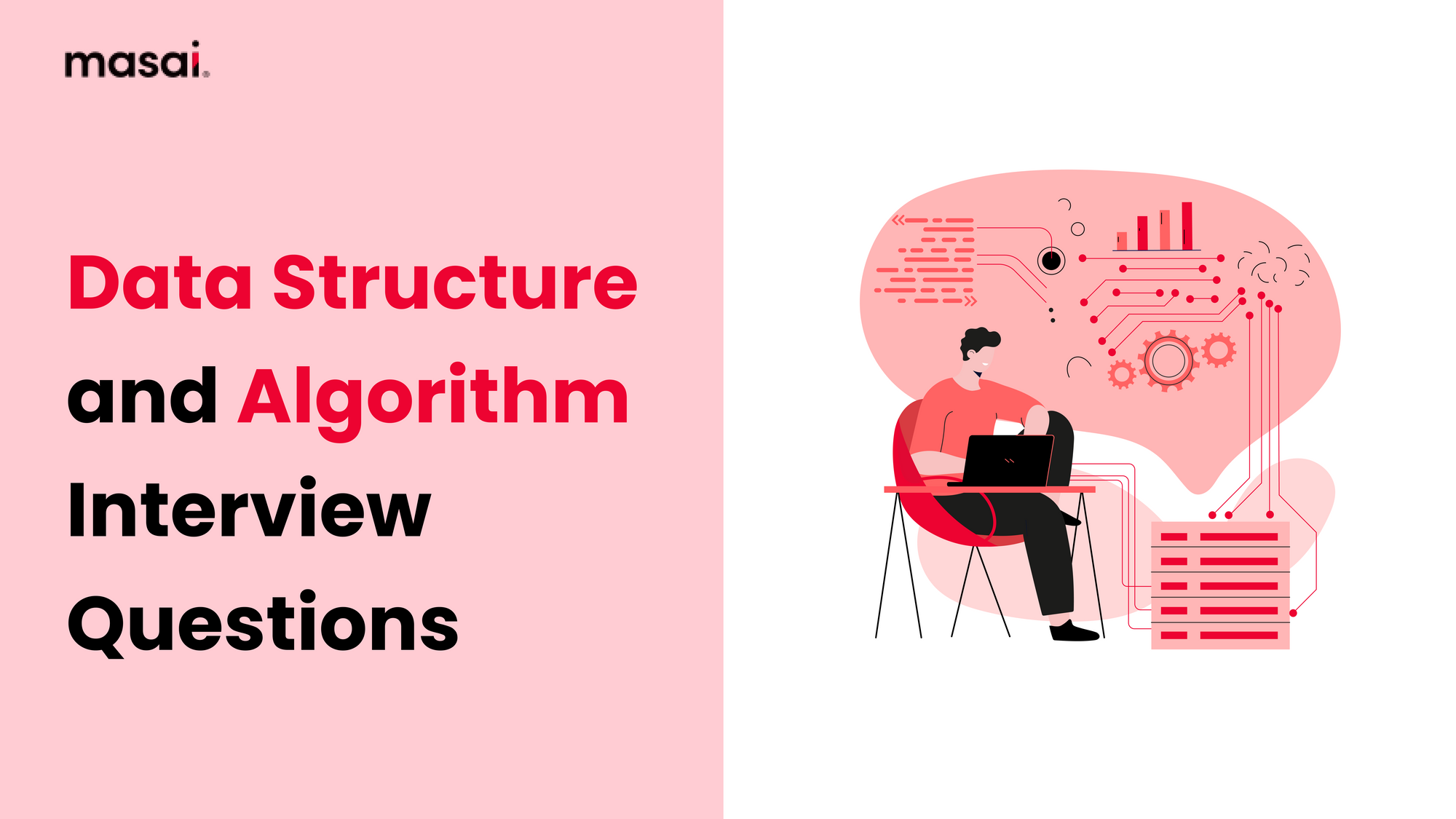
Introduction
Are you applying for a job for a coding, programming, or software development position? Preparing yourself with skillful answers to questions for data structure and algorithm interviews helps you cut the ice. Top data science courses in India will help you quickly navigate the complex interview process and impress your employer.
A solid understanding of the varied concepts of data structures and algorithms is crucial to enhancing your problem-solving skills. Data structure and algorithm interview questions will evaluate your coding and problem-solving skills with clarity of thought by the answers you provide, including showcasing your hands-on experience, if any.
Here, we take you through a series of the leading interview preparation questions on data structures and algorithms.
What are Data Structures, and Why Would You Create Them?
Organizing data mechanically or logically within a program is called a data structure. How you organize data determines how a particular program performs. There are several types of data structures intended for different purposes. Designing the code depends on the way the data is structured. If the data is not structured correctly or stored efficiently, the performance of the code will also not be effective.
A data structure serves a series of functions in a program, ensuring every line of code performs the specific function to the optimum. It helps you identify and fix problems with the code and create a more organized code base.
What is the Difference Between File Structure and Storage Structure?
Data stored in secondary or auxiliary memory, such as pen drives or hard disks, remains intact until manually deleted. It's a data representation of a file structure. In the storage structure, the data is stored in the main memory like the RAM and is deleted immediately after the function uses this data and executes it
How Do You Identify if Two Integers Have Opposite Signs?
Bit manipulation allows you to work with bits instead of abstractions to quickly detect and correct algorithms, data compression, and optimization. You can use bit-wise operations to work with individual bits that form the minor units of data.
When you have two integers with opposite signs, their significant bits will be different, i.e., the significant bit of any negative number will be one. For the positive number, it’ll be zero. Applying the bit-wise operator to these two integers will give you a negative number. Hence, the XOR operator will return less than zero, signifying the two numbers have opposite signs.
What is a Stack Data Structure, and What Are Its Applications?
A stack data structure helps you represent an application's state at a specific time. It includes a series of items that accumulate at the top, which are removed. The linear data structure follows a specific order in which you perform operations. The standard orders are LIFO (Last In First Out) and FILO (First In Last Out). The elements added last to the stack will come out first.
The common applications of the stack data structure are:
- Temporary storage during recursive operations
- Redo and Undo operations in doc editors
- Reversing a string
- Parenthesis matching
- Postfix to Infix Expressions
- Function calls order
What is the Difference Between an Array and a Stack?
This is one of the popular data structure and algorithm interview questions, and your answer will demonstrate how well you understand the two diverse concepts. Firstly, stacks and arrays store data in two different ways; stacks store different data types, whereas arrays store the same data type. Stacks can change their size as and when elements are added or removed, while arrays don’t change in size. The LIFO principle applies to stacks, while a specific variable with its index is needed to pick an element in an array.
Give a thorough explanation of a queue data structure, its application, and the different operations available.
A queue is a linear data structure where you can systematically store items. These items are added to the queue at the rear end and are removed from the front when the queue is full. This structure is commonly used in situations that demand holding items for an extended period. The queue data structure is commonly used in job scheduling operations and call management services.
The operations available in a queue data structure are:
- Enqueue to add an element or an item to the rear end that returns the overflow condition when the queue is full
- Dequeue removes an item from the front end that returns the underflow condition when the queue is empty
- isEmpty that returns true only if the queue is empty
- Rear that returns the rear-end element but doesn’t remove it
- Front that returns the front element but doesn’t remove it
- Size that returns the size of the queue
What is the Difference Between a Linear Data Structure and a Hierarchical Data Structure?
Both types of data structures describe the relationships between the types of data. However, they differ in how the data interacts with each other. In linear data structures, data is organized in a single-level sequence. In a hierarchical data structure, data is organized in a multi-level configuration.
What is Binary Search Tree Data Structure, and What are its Applications?
This data structure stores items in a sorted order where each node stores a key and a value. The key helps you access the item where the value determines if the item is present. The key is commonly an integer, a floating point number, a character string, or a combination of these. A node is added to the key to access the item stored in that particular node. The binary tree has a specific order of elements like:
Elements in the left sub-tree of a node should have a value that is less than or equal to the parent node’s value.
Elements in the right sub-tree of a node should have a value greater than or equal to the parent node’s value.
Its typical applications are:
- Used for indexing and multi-level indexing
- Used for implementing various search algorithms
- Used for organizing a sorted stream of data
The Takeaway
This post covers the top questions asked in data structure and algorithm interviews. These should help you immensely with interview preparation and give a complete picture to the employer regarding your skills and abilities to drive home results. Understand that these questions are critical throughout all interviews and levels, and they determine whether you’re hired or not.
FAQs
Why are data structures and algorithms important in technical interviews?
Data structures and algorithms play a vital role in computer science and in solving complex programming issues efficiently. A technical interview helps assess a candidate’s skills regarding problem-solving, algorithmic thinking, and the ability to craft effective solutions.
List the most commonly tested data structures and algorithms.
Data structures like linked lists, arrays, queues, stacks, and graphs are the most commonly tested structures and algorithms. Algorithms like sorting, searching, and dynamic programming are used frequently.
What is the method to approach studying data structures and algorithms?
You can begin by building a robust foundation in the data structures like arrays, queues, stacks, etc. Next, you can head to more complex structures like trees and graphs. The point is to understand each algorithm's critical operations and time constraints. It pays to implement the techniques from scratch and solve problems accordingly.
How to handle difficult and unfamiliar issues?
It’s advisable to break the problem into smaller parts and check for similarities with previously solved problems. You can also simplify the problem solving with a more accessible version first.
Q. Why is it important to know the theory behind each data structure and algorithm?
It’s a known fact that practical implementation of the issue is crucial. However, understanding the theory behind every data structure and algorithm helps you make better and more informed decisions regarding which to use in a specific situation and optimize the usage.