Handling Command Line Arguments in Java Applications
Applications are frequently run using arguments from the command line. We'll look at how to handle command-line arguments in Java in this blog.
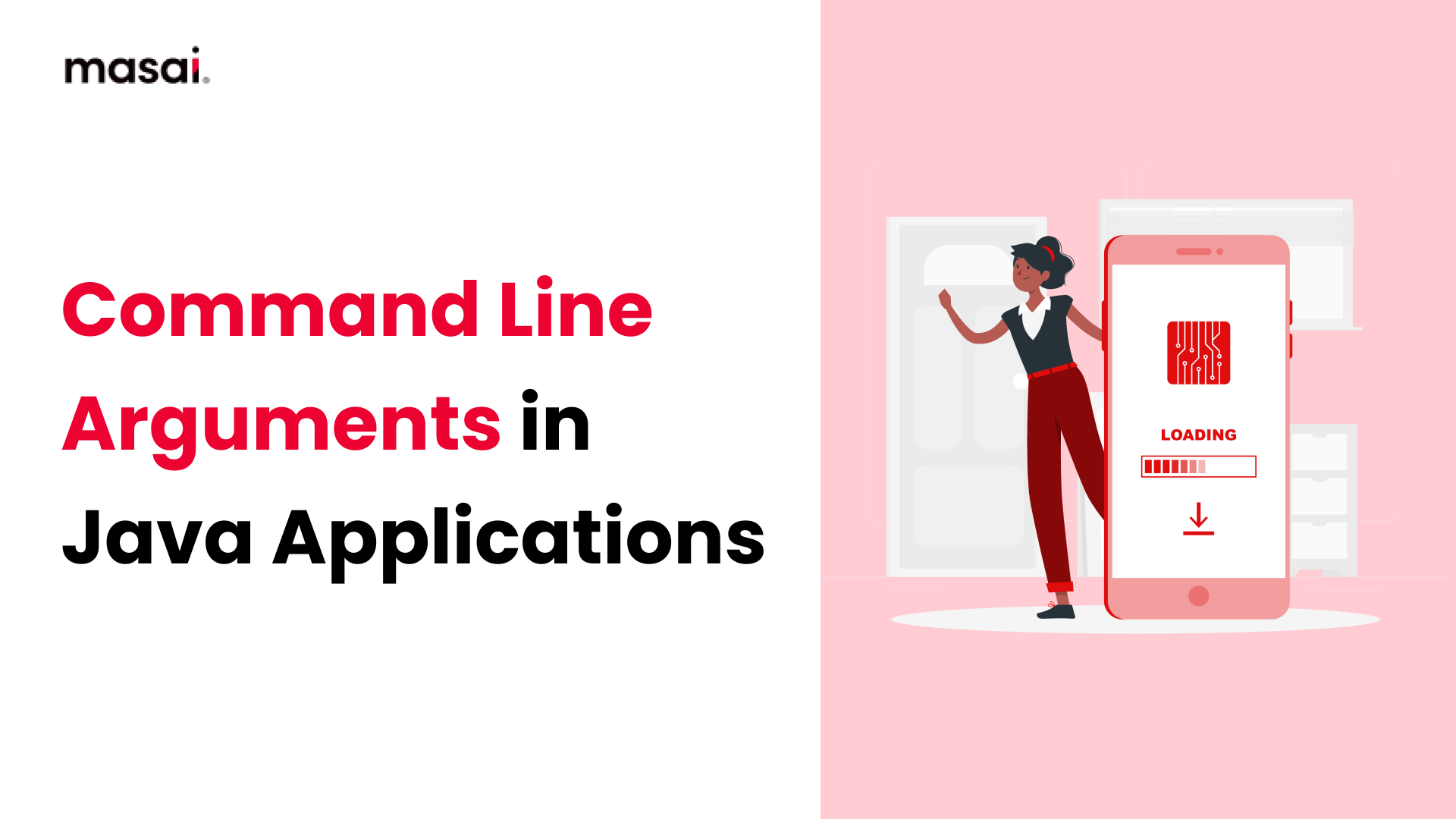
Introduction
Java applications often need to interact with users and external systems through various means. One fundamental way to provide input to a Java program during runtime is by utilizing command-line arguments. These arguments are crucial in tailoring program behavior and enhancing user experience. This guide will delve into the world of command-line arguments, explore their significance, and learn how to handle them effectively in Java applications.
Understanding Command Line Arguments
When a program is run from the command line or terminal, it receives input parameters known as command line arguments. Command line arguments are supplied when the program is launched instead of user input gathered during program runtime. Users can alter a program's behavior without changing its original code.
Command line arguments in Java are a way to send data to a program's main function. This allows web developers to design flexible applications to set and control users or other systems.
The syntax for Command Line Arguments
You must follow a specific syntax to pass command line arguments to a Java program. When executing a Java application from the command line, the general syntax is as follows:
java YourProgramClassName arg1 arg2 arg3 ...
Here, YourProgramClassName should be replaced with the name of your Java class containing the main method, and arg1, arg2, etc., represent the individual command line arguments you want to pass.
For instance, consider a Java program named Calculator that performs basic arithmetic operations. To provide two numbers, 5 and 10, as input parameters to the program, you would run the following:
java Calculator 5+10
Accessing Command Line Arguments
Accessing command line arguments in a Java program is straightforward. The main method of your Java class includes a String[] args parameter, which serves as an array to hold the command line arguments.
Here's a simple code snippet illustrating how to access and print command line arguments:
java
public class CommandLineExample {
public static void main(String[] args) {
for (String arg : args) {
System.out.println("Argument: " arg);
}
}
}
Processing Command Line Arguments
Processing command line arguments involves interpreting and utilizing the provided input parameters to customize program behavior. This can include tasks such as performing calculations, reading files, or configuring settings based on the arguments received.
Let's extend our previous example of the Calculator program. We can modify the program to parse the command line arguments as numbers and perform arithmetic operations:
java
public class Calculator {
public static void main(String[] args) {
if (args.length != 3) {
System.out.println("Usage: java Calculator num1 operator num2");
return;
}
double num1 = Double.parseDouble(args[0]);
String operator = args[1];
double num2 = Double.parseDouble(args[2]);
double result = 0.0;
switch (operator) {
case " ":
result = num1 num2;
break;
case "-":
result = num1 - num2;
break;
case "*":
result = num1 * num2;
break;
case "/":
if (num2 != 0) {
result = num1 / num2;
} else {
System.out.println("Error: Division by zero");
return;
}
break;
default:
System.out.println("Error: Invalid operator");
return;
}
System.out.println("Result: " result);
}
} Â
Best Practices for Command Line Argument Handling
When working with command line arguments in Java applications, it's essential to follow these best practices:
Clear Documentation: Provide concise and comprehensive documentation for your program's command line options and usage. Users should clearly understand how to provide arguments and what they signify.
Error Handling: Implement robust error handling to address cases where incorrect or insufficient arguments are provided. Provide informative error messages to guide users toward correct usage.
Third-party Libraries: Consider using third-party libraries like Apache Commons CLI to simplify command-line argument parsing and handling. These libraries offer advanced features and options for more complex scenarios.
Advanced Topics
As your Java programming skills evolve, you might encounter more advanced command-line argument-handling scenarios:
Named Arguments and Flags: Instead of relying solely on the order of arguments, you can implement named arguments (also known as options) and flags. This allows users to provide arguments in any order and enhances the user experience.
Complex Scenarios: Some applications require intricate command line interfaces with nested subcommands, hierarchical options, and custom help messages. Understanding these advanced scenarios can elevate your command-line argument-handling skills.
Integration with Logging and Debugging: Integrate command line argument handling with your application's logging and debugging mechanisms. This can help you diagnose issues and provide detailed insights when troubleshooting.
Pitfalls to Avoid
When working with command line arguments, be mindful of these common pitfalls:
Insufficient Validation: Failing to validate input parameters can lead to unexpected program behavior or security vulnerabilities. Always validate and sanitize user input before processing.
Inadequate Error Handling: Neglecting proper error handling can result in confusing or misleading error messages for users. Implement meaningful error messages to guide users toward correct usage.
Complex Argument Structures: While advanced argument structures can provide flexibility, they can also increase complexity and make the program less user-friendly. Strike a balance between flexibility and simplicity.
Conclusion
Mastering the art of handling command line arguments is a fundamental skill for Java developers. You can create versatile and user-friendly applications by understanding the syntax, accessing and processing arguments, and implementing best practices. Whether building a simple calculator or a sophisticated configuration utility, command line arguments empower you to build dynamic and customizable Java programs. Embrace the power of command line arguments and unlock new possibilities in your Java development journey.
FAQs
What are command-line arguments in Java applications?
When a Java program is run from the command line or terminal, it receives input parameters known as command-line arguments. They enable users to adjust the behavior of a program without changing its code.
Why are command-line arguments important in Java programming?
Java programs can be flexible and customized thanks to command-line arguments. They allow users to enter data while the program runs, allowing for greater scenario flexibility.
What's the syntax for passing command-line arguments to a Java program?
Use the syntax: “java YourProgramClassName arg1 arg2 arg3” to pass command-line arguments. Replace “arg1, arg2,...” with the real arguments and “YourProgramClassName” with the name of your class.
How can developers integrate command-line argument handling with logging and debugging?
To detect problems and give thorough understanding during troubleshooting, command-line argument handling should be integrated with logging and debugging systems.
What are some best practices for handling command-line arguments in Java applications?
Best practices include considering third-party libraries like Apache Commons CLI for sophisticated parsing, providing explicit explanations of choices, and having robust error handling for invalid parameters.